381. Insert Delete GetRandom O(1) - Duplicates allowed
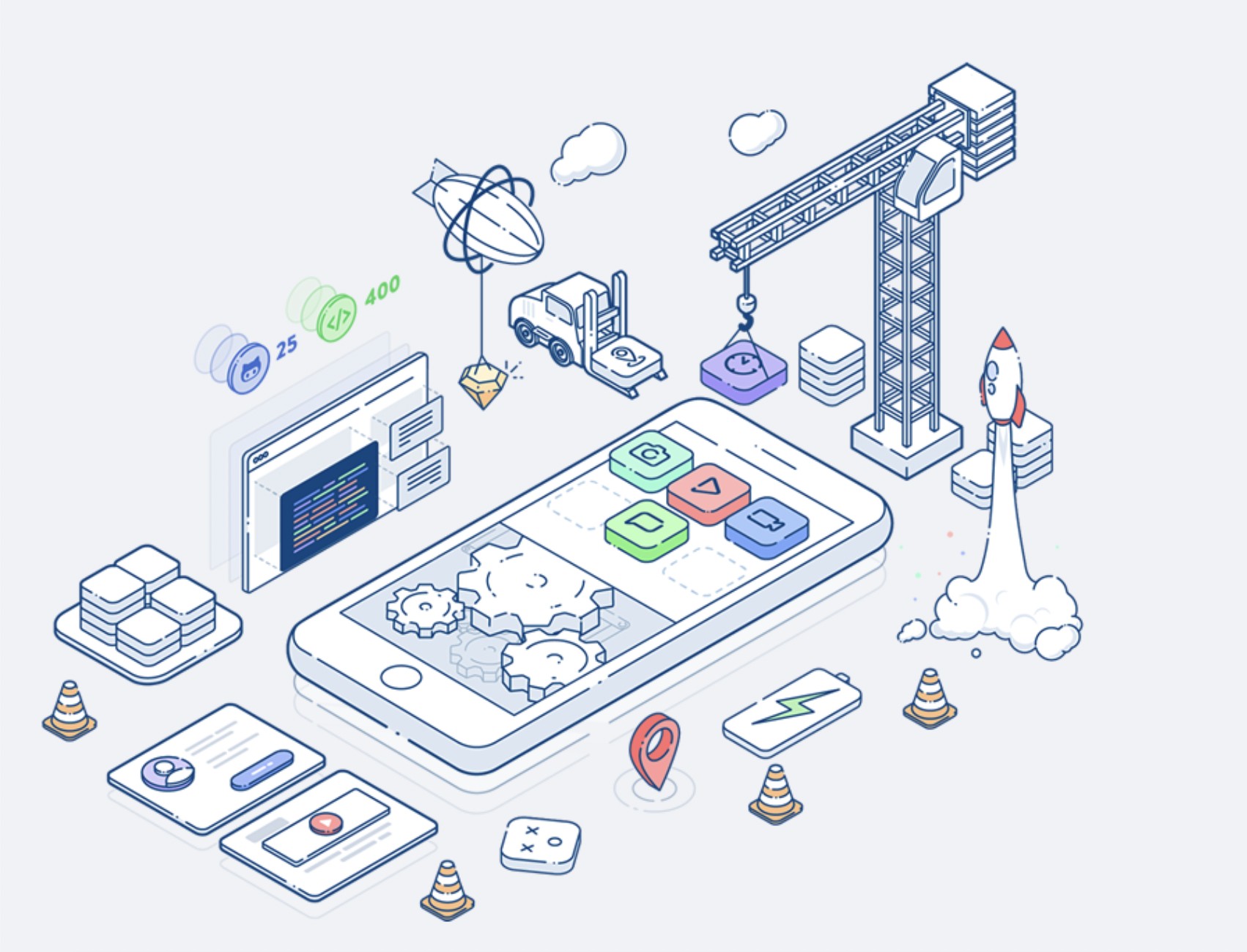
Design a data structure that supports all following operations in average O(1) time.
Note: Duplicate elements are allowed. insert(val): Inserts an item val to the collection. remove(val): Removes an item val from the collection if present. getRandom: Returns a random element from current collection of elements. The probability of each element being returned is linearly related to the number of same value the collection contains. Example:
// Init an empty collection.RandomizedCollection collection = new RandomizedCollection();// Inserts 1 to the collection. Returns true as the collection did not contain 1.collection.insert(1);// Inserts another 1 to the collection. Returns false as the collection contained 1. Collection now contains [1,1].collection.insert(1);// Inserts 2 to the collection, returns true. Collection now contains [1,1,2].collection.insert(2);// getRandom should return 1 with the probability 2/3, and returns 2 with the probability 1/3.collection.getRandom();// Removes 1 from the collection, returns true. Collection now contains [1,2].collection.remove(1);// getRandom should return 1 and 2
思路: 那么查询还是直接操作ArrayList没有难度 而插入的时候,是插入到位置集合也没有难度 删除的时候,选择Stack,需要保证出栈的顺序有保障
class RandomizedCollection { //用来索引下在ArrayList中的关系,做到快速定位 private HashMap> locations; //存储原始数据 private ArrayList list; private Random random; //为了排序,控制栈的 ArrayList tmpForStack; /** Initialize your data structure here. */ public RandomizedCollection() { this.locations = new HashMap>(); this.list = new ArrayList(); this.random = new Random(); this.tmpForStack = new ArrayList(); } /** Inserts a value to the collection. Returns true if the collection did not already contain the specified element. */ public boolean insert(int val) { boolean flag = false; if(locations.containsKey(val) == false){ locations.put(val, new Stack()); } if(locations.get(val).isEmpty()) flag = true; locations.get(val).push(list.size()); list.add(val); return flag; } /** Removes a value from the collection. Returns true if the collection contained the specified element. */ public boolean remove(int val) { if(locations.containsKey(val) == false || locations.get(val).isEmpty()) return false; int tmpLocation = locations.get(val).pop(); if(tmpLocation != list.size() - 1){ int lastVal = list.get(list.size() -1); list.set(tmpLocation, lastVal); locations.get(lastVal).pop(); tmpForStack.clear(); while(locations.get(lastVal).isEmpty() == false && locations.get(lastVal).peek() > tmpLocation){ tmpForStack.add(locations.get(lastVal).pop()); } locations.get(lastVal).push(tmpLocation); for(int tval:tmpForStack) locations.get(lastVal).push(tval); } list.remove(list.size() - 1); return true; } /** Get a random element from the collection. */ public int getRandom() { return list.get(random.nextInt(list.size())); }}/** * Your RandomizedCollection object will be instantiated and called as such: * RandomizedCollection obj = new RandomizedCollection(); * boolean param_1 = obj.insert(val); * boolean param_2 = obj.remove(val); * int param_3 = obj.getRandom(); */
版权声明:本文内容由网络用户投稿,版权归原作者所有,本站不拥有其著作权,亦不承担相应法律责任。如果您发现本站中有涉嫌抄袭或描述失实的内容,请联系我们jiasou666@gmail.com 处理,核实后本网站将在24小时内删除侵权内容。
暂时没有评论,来抢沙发吧~