C#反射Helper
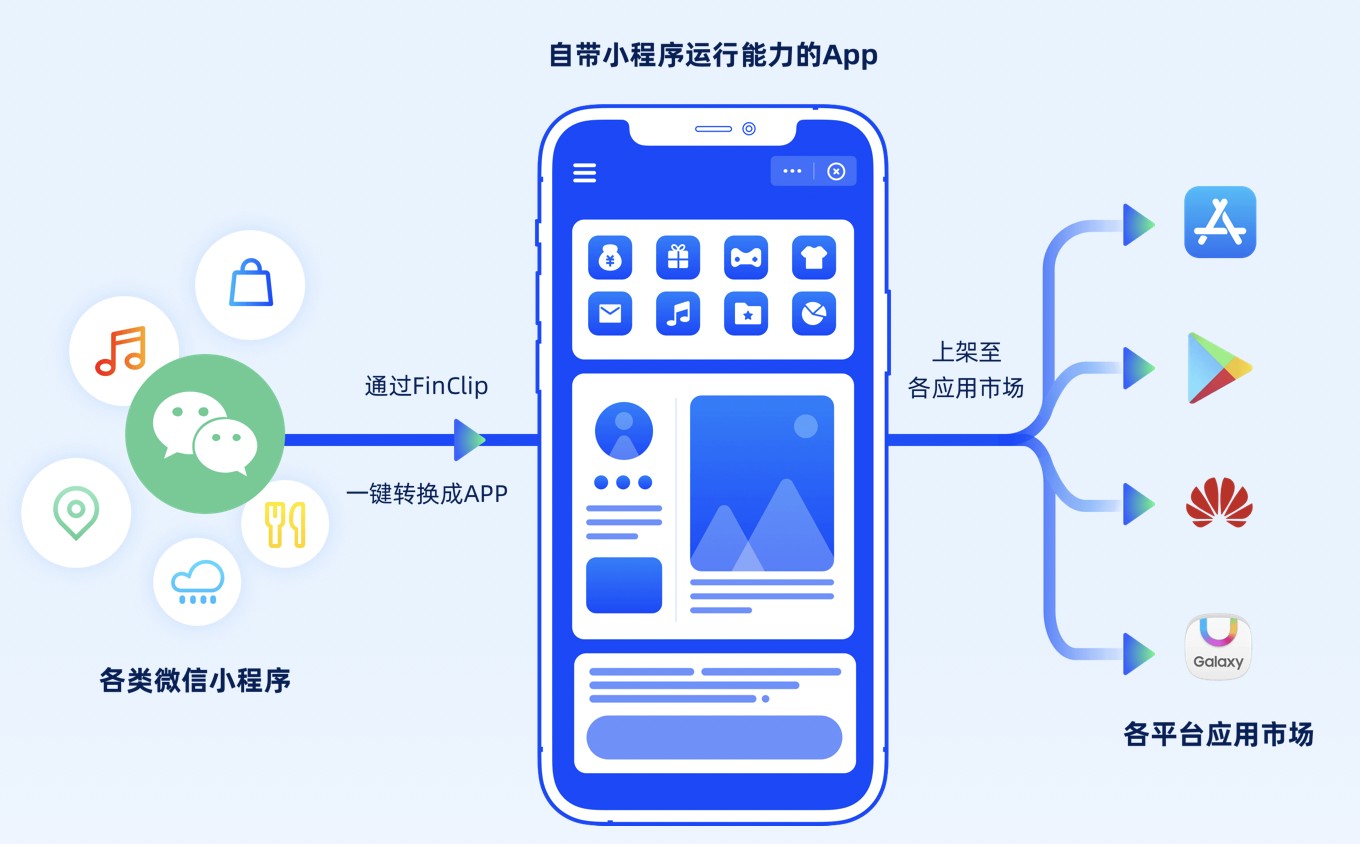
//反射 操作 public static class Reflection { #region GetDescription(获取类型描述) /// /// 获取类型描述,使用设置描述 /// /// 类型 public static string GetDescription() => GetDescription(Common.GetType()); /// /// 获取类型成员描述,使用设置描述 /// /// 类型 /// 成员名称 public static string GetDescription(string memberName) => GetDescription(Common.GetType(), memberName); /// /// 获取类型成员描述,使用设置描述 /// /// 类型 /// 成员名称 public static string GetDescription(Type type, string memberName) { if (type == null) return string.Empty; return memberName.IsEmpty() ? string.Empty : GetDescription(type.GetTypeInfo().GetMember(memberName).FirstOrDefault()); } /// /// 获取类型成员描述,使用设置描述 /// /// 成员 public static string GetDescription(MemberInfo member) { if (member == null) return string.Empty; return member.GetCustomAttribute() is DescriptionAttribute attribute ? attribute.Description : member.Name; } #endregion #region GetDisplayName(获取类型显示名称) /// /// 获取类型显示名称,使用设置显示名称 /// /// 类型 /// public static string GetDisplayName() => GetDisplayName(Common.GetType()); /// /// 获取类型成员显示名称,,使用或设置显示名称 /// /// 成员 /// private static string GetDisplayName(MemberInfo member) { if (member == null) return string.Empty; if (member.GetCustomAttribute() is DisplayAttribute displayAttribute) return displayAttribute.Description; if (member.GetCustomAttribute() is DisplayNameAttribute displayNameAttribute) return displayNameAttribute.DisplayName; return string.Empty; } #endregion #region GetDisplayNameOrDescription(获取显示名称或类型描述) /// /// 获取类型显示名称或描述,使用设置描述,使用设置显示名称 /// /// 类型 /// public static string GetDisplayNameOrDescription() { return GetDisplayNameOrDescription(Common.GetType()); } /// /// 获取类型显示名称或成员描述,使用设置描述,使用或设置显示名称 /// /// 成员 /// public static string GetDisplayNameOrDescription(MemberInfo member) { var result = GetDisplayName(member); return string.IsNullOrWhiteSpace(result) ? GetDescription(member) : result; } #endregion #region FindTypes(查找类型列表) /// /// 查找类型列表 /// /// 查找类型 /// 待查找的程序集列表 /// public static List FindType(params Assembly[] assemblies) { var findType = typeof(TFind); return FindTypes(findType, assemblies); } /// /// 查找类型列表 /// /// 查找类型 /// 待查找的程序集列表 /// public static List FindTypes(Type findType, params Assembly[] assemblies) { var result = new List(); foreach (var assembly in assemblies) { result.AddRange(GetTypes(findType, assembly)); } return result.Distinct().ToList(); } /// /// 获取类型列表 /// /// 查找类型 /// 待查找的程序集 /// private static List GetTypes(Type findType, Assembly assembly) { var result = new List(); if (assembly == null) return result; Type[] types; try { types = assembly.GetTypes(); } catch (ReflectionTypeLoadException) { return result; } foreach (var type in types) AddType(result, findType, type); return result; } /// /// 添加类型 /// /// 类型列表 /// 查找类型 /// 类型 private static void AddType(List result, Type findType, Type type) { if (type.IsInterface || type.IsAbstract) return; if (findType.IsAssignableFrom(type) == false && MatchGeneric(findType, type) == false) return; result.Add(type); } /// /// 泛型匹配 /// /// 查找类型 /// 类型 /// private static bool MatchGeneric(Type findType, Type type) { if (findType.IsGenericTypeDefinition == false) return false; var definition = findType.GetGenericTypeDefinition(); foreach (var implementedInterface in type.FindInterfaces((filiter, criteria) => true, null)) { if (implementedInterface.IsGenericType == false) continue; return definition.IsAssignableFrom(implementedInterface.GetGenericTypeDefinition()); } return false; } #endregion #region GetInstancesByInterface(获取实现了接口的所有实例) /// /// 获取实现了接口的所有实例 /// /// 接口类型 /// 在该程序集中查找 public static List GetInstancesByInterface(Assembly assembly) { var typeInterface = typeof(TInterface); return assembly.GetTypes() .Where( t => typeInterface.GetTypeInfo().IsAssignableFrom(t) && t != typeInterface && t.GetTypeInfo().IsAbstract == false) .Select(t => CreateInstance(t)) .ToList(); } #endregion #region CreateInstance(动态创建实例) /// /// 动态创建实例 /// /// 目标类型 /// 类型 /// 传递给构造函数的参数 public static T CreateInstance(Type type, params object[] parameters) => Conv.To(Activator.CreateInstance(type, parameters)); /// /// 动态创建实例 /// /// 目标类型 /// 类名,包括命名空间,如果类型不处于当前执行程序集中,需要包含程序集名,范例:Test.Core.Test2,Test.Core /// 传递给构造函数的参数 public static T CreateInstance(string className, params object[] parameters) { var type = Type.GetType(className) ?? Assembly.GetCallingAssembly().GetType(className); return CreateInstance(type, parameters); } #endregion #region GetAssembly(获取程序集) /// /// 获取程序集 /// /// 程序集名称 /// public static Assembly GetAssembly(string assemblyName) => Assembly.Load(new AssemblyName(assemblyName)); #endregion #region GetAssemblies(从目录获取所有程序集) /// /// 从目录获取所有程序集 /// /// 目录绝对路径 /// public static List GetAssemblies(string directoryPath) { return Directory.GetFiles(directoryPath, "*.*", SearchOption.AllDirectories) .ToList() .Where(t => t.EndsWith(".exe") || t.EndsWith(".dll")) .Select(path => Assembly.Load(new AssemblyName(path))) .ToList(); } #endregion #region GetCurrentAssemblyName(获取当前程序集名称) /// /// 获取当前程序集名称 /// public static string GetCurrentAssemblyName() => Assembly.GetCallingAssembly().GetName().Name; #endregion #region GetAttribute(获取特性信息) /// /// 获取特性信息 /// /// 泛型特性 /// 元数据 public static TAttribute GetAttribute(MemberInfo memberInfo) where TAttribute : Attribute { return (TAttribute)memberInfo.GetCustomAttributes(typeof(TAttribute), false).FirstOrDefault(); } #endregion #region GetAttributes(获取特性信息数据) /// /// 获取特性信息数组 /// /// 泛型特性 /// 元数据 /// public static TAttribute[] GetAttributes(MemberInfo memberInfo) where TAttribute : Attribute { return Array.ConvertAll(memberInfo.GetCustomAttributes(typeof(TAttribute), false), x => (TAttribute)x); } #endregion #region GetPropertyInfo(获取属性信息) /// /// 获取属性信息 /// /// 类型 /// 属性名 /// 存在时返回PropertyInfo,不存在时返回null public static PropertyInfo GetPropertyInfo(Type type, string propertyName) => type.GetProperties().FirstOrDefault(p => p.Name.Equals(propertyName)); #endregion #region IsBool(是否布尔类型) /// /// 是否布尔类型 /// /// 成员 /// public static bool IsBool(MemberInfo member) { if (member == null) return false; switch (member.MemberType) { case MemberTypes.TypeInfo: return member.ToString() == "System.Boolean"; case MemberTypes.Property: return IsBool((PropertyInfo)member); } return false; } /// /// 是否布尔类型 /// /// 属性 /// public static bool IsBool(PropertyInfo property) { return property.PropertyType == typeof(bool) || property.PropertyType == typeof(bool?); } #endregion #region IsEnum(是否枚举类型) /// /// 是否枚举类型 /// /// 成员 /// public static bool IsEnum(MemberInfo member) { if (member == null) { return false; } switch (member.MemberType) { case MemberTypes.TypeInfo: return ((TypeInfo)member).IsEnum; case MemberTypes.Property: return IsEnum((PropertyInfo)member); } return false; } /// /// 是否枚举类型 /// /// 属性 /// public static bool IsEnum(PropertyInfo property) { if (property.PropertyType.GetTypeInfo().IsEnum) { return true; } var value = Nullable.GetUnderlyingType(property.PropertyType); if (value == null) { return false; } return value.GetTypeInfo().IsEnum; } #endregion #region IsDate(是否日期类型) /// /// 是否日期类型 /// /// 成员 /// public static bool IsDate(MemberInfo member) { if (member == null) { return false; } switch (member.MemberType) { case MemberTypes.TypeInfo: return member.ToString() == "System.DateTime"; case MemberTypes.Property: return IsDate((PropertyInfo)member); } return false; } /// /// 是否日期类型 /// /// 属性 /// public static bool IsDate(PropertyInfo property) { if (property.PropertyType == typeof(DateTime)) { return true; } if (property.PropertyType == typeof(DateTime?)) { return true; } return false; } #endregion #region IsInt(是否整型) /// /// 是否整型 /// /// 成员 /// public static bool IsInt(MemberInfo member) { if (member == null) { return false; } switch (member.MemberType) { case MemberTypes.TypeInfo: return member.ToString() == "System.Int32" || member.ToString() == "System.Int16" || member.ToString() == "System.Int64"; case MemberTypes.Property: return IsInt((PropertyInfo)member); } return false; } /// /// 是否整型 /// /// 成员 /// public static bool IsInt(PropertyInfo property) { if (property.PropertyType == typeof(int)) { return true; } if (property.PropertyType == typeof(int?)) { return true; } if (property.PropertyType == typeof(short)) { return true; } if (property.PropertyType == typeof(short?)) { return true; } if (property.PropertyType == typeof(long)) { return true; } if (property.PropertyType == typeof(long?)) { return true; } return false; } #endregion #region IsNumber(是否数值类型) /// /// 是否数值类型 /// /// 成员 /// public static bool IsNumber(MemberInfo member) { if (member == null) { return false; } if (IsInt(member)) { return true; } switch (member.MemberType) { case MemberTypes.TypeInfo: return member.ToString() == "System.Double" || member.ToString() == "System.Decimal" || member.ToString() == "System.Single"; case MemberTypes.Property: return IsNumber((PropertyInfo)member); } return false; } /// /// 是否数值类型 /// /// 属性 /// public static bool IsNumber(PropertyInfo property) { if (property.PropertyType == typeof(double)) { return true; } if (property.PropertyType == typeof(double?)) { return true; } if (property.PropertyType == typeof(decimal)) { return true; } if (property.PropertyType == typeof(decimal?)) { return true; } if (property.PropertyType == typeof(float)) { return true; } if (property.PropertyType == typeof(float?)) { return true; } return false; } #endregion #region IsCollection(是否集合) /// /// 是否集合 /// /// 类型 public static bool IsCollection(Type type) => type.IsArray || IsGenericCollection(type); #endregion #region IsGenericCollection(是否泛型集合) /// /// 是否泛型集合 /// /// 类型 public static bool IsGenericCollection(Type type) { if (!type.IsGenericType) return false; var typeDefinition = type.GetGenericTypeDefinition(); return typeDefinition == typeof(IEnumerable<>) || typeDefinition == typeof(IReadOnlyCollection<>) || typeDefinition == typeof(IReadOnlyList<>) || typeDefinition == typeof(ICollection<>) || typeDefinition == typeof(IList<>) || typeDefinition == typeof(List<>); } #endregion #region GetPublicProperties(获取公共属性列表) /// /// 获取公共属性列表 /// /// 实例 public static List- GetPublicProperties(object instance) { var properties = instance.GetType().GetProperties(); return properties.ToList().Select(t => new Item(t.Name, t.GetValue(instance))).ToList(); } #endregion #region GetTopBaseType(获取顶级基类) /// /// 获取顶级基类 /// /// 类型 /// public static Type GetTopBaseType() { return GetTopBaseType(typeof(T)); } /// /// 获取顶级基类 /// /// 类型 /// public static Type GetTopBaseType(Type type) { if (type == null) { return null; } if (type.IsInterface) { return type; } if (type.BaseType == typeof(object)) { return type; } return GetTopBaseType(type.BaseType); } #endregion #region IsGenericAssignableFrom(判断当前泛型类型是否可由指定类型的实例填充) /// /// 判断当前泛型类型是否可由指定类型的实例填充 /// /// 泛型类型 /// 指定类型 public static bool IsGenericAssignableFrom(Type genericType, Type type) { Check.NotNull(genericType, nameof(genericType)); Check.NotNull(type, nameof(type)); if (!genericType.IsGenericType) { throw new ArgumentException("该功能只支持泛型类型的调用,非泛型类型可使用 IsAssignableFrom 方法。"); } var allOthers = new List() { type }; if (genericType.IsInterface) { allOthers.AddRange(type.GetInterfaces()); } foreach (var other in allOthers) { var cur = other; while (cur != null) { if (cur.IsGenericType) { cur = cur.GetGenericTypeDefinition(); } if (cur.IsSubclassOf(genericType) || cur == genericType) { return true; } cur = cur.BaseType; } } return false; } #endregion #region GetElementType(获取元素类型) /// /// 获取元素类型。如果是集合,返回集合的元素类型 /// /// 类型 public static Type GetElementType(Type type) { if (IsCollection(type) == false) return type; if (type.IsArray) return type.GetElementType(); var genericArgumentsTypes = type.GetTypeInfo().GetGenericArguments(); if (genericArgumentsTypes == null || genericArgumentsTypes.Length == 0) throw new ArgumentException("泛型类型参数不能为空"); return genericArgumentsTypes[0]; } #endregion }
#region IsEmpty(是否为空) /// /// 判断 字符串 是否为空、null或空白字符串 /// /// 数据 public static bool IsEmpty(this string value) => string.IsNullOrWhiteSpace(value); #endregion #region SafeString(安全转换为字符串) /// /// 安全转换为字符串,去除两端空格,当值为null时返回"" /// /// 输入值 public static string SafeString(this object input) => input == null ? string.Empty : input.ToString().Trim(); #endregion
版权声明:本文内容由网络用户投稿,版权归原作者所有,本站不拥有其著作权,亦不承担相应法律责任。如果您发现本站中有涉嫌抄袭或描述失实的内容,请联系我们jiasou666@gmail.com 处理,核实后本网站将在24小时内删除侵权内容。
暂时没有评论,来抢沙发吧~