设计模式复习-迭代器模式
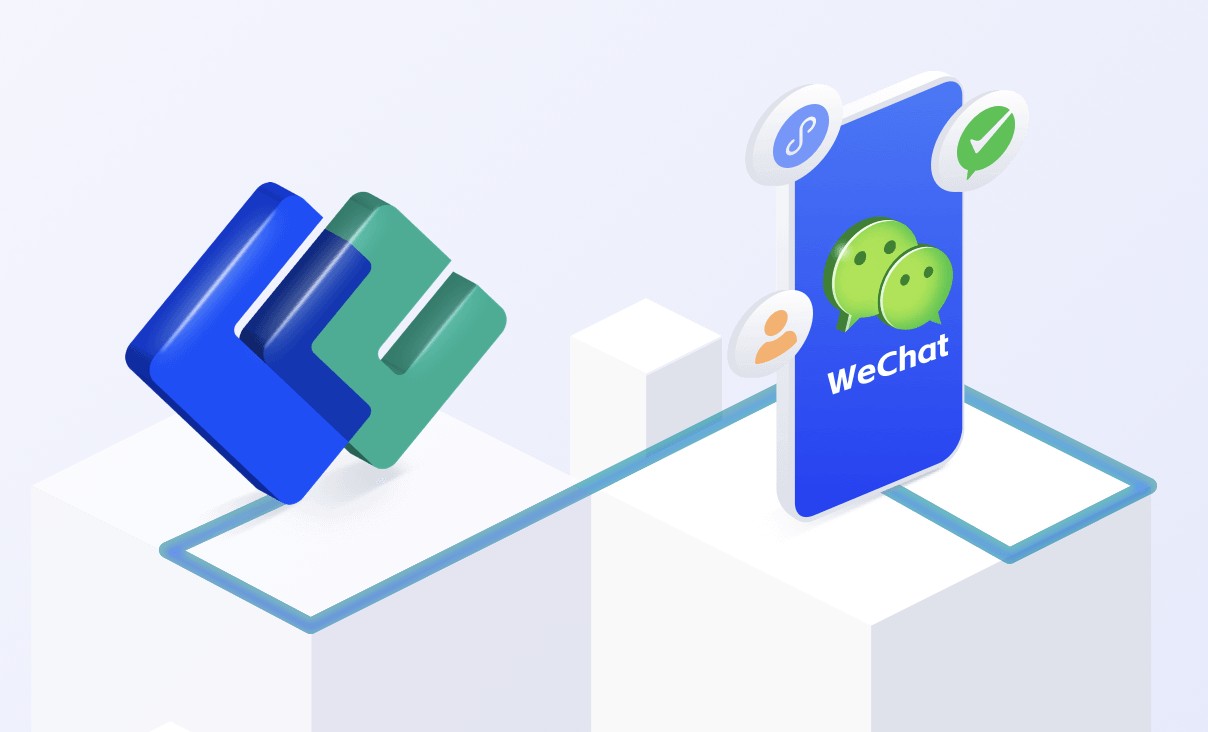
Iterator.H#pragma once#include #include using namespace std;/*设计模式-迭代器模式(Iterator)提供一种方法顺序访问一个聚合对象中的各个元素,而不暴露该对象内部表示。(现在好多语言都已经内置实现了这个功能了,所以实际用途不大,但是建议写一下,实现过程价值远远大于使用价值。)*/class Iterator{//迭代器抽象类public: virtual void * First() = 0; virtual void * Next() = 0; virtual BOOL IsDone() = 0; virtual void * CurrentItem() = 0;};class CAggregate {//聚集抽象类public: virtual Iterator * CreateIterator() = 0; virtual void Insert(void * const pNode) = 0; virtual void Remove(void * const pNode) = 0;};class CConcreteAggregate :public CAggregate {//具体聚集类public: listmpItems; CConcreteAggregate(); void Clear(); Iterator * CreateIterator(); void Insert(void * const pNode); void Remove(void * const pNode);};class CConcreteIterator : public Iterator {//具体的迭代器类private: CConcreteAggregate *mpAggregate = NULL; int mnCurrent = 0;public: CConcreteIterator(CConcreteAggregate * pAggregate); void * First(); void * Next(); BOOL IsDone(); void * CurrentItem();};Iterator.CPP#include "stdafx.h"#include "Iterator.h"CConcreteAggregate::CConcreteAggregate() { mpItems.clear();}void CConcreteAggregate::Clear() { for each(auto i in mpItems) { delete i; }}Iterator * CConcreteAggregate::CreateIterator() { return new CConcreteIterator(this);}void CConcreteAggregate::Insert(void * const pNode) { mpItems.push_back(pNode);}void CConcreteAggregate::Remove(void * const pNode) { mpItems.remove(pNode);}CConcreteIterator::CConcreteIterator(CConcreteAggregate * pAggregate) { mpAggregate = pAggregate; mnCurrent = 0;}void * CConcreteIterator::First() { return mpAggregate->mpItems.size() == 0 ? NULL : *(mpAggregate->mpItems.begin());}void * CConcreteIterator::Next() { if (IsDone()) return NULL; int nSubscript = 0; mnCurrent++; for each(auto i in mpAggregate->mpItems) { if (nSubscript++ == mnCurrent + 1) { return i; } }}BOOL CConcreteIterator::IsDone() { return mnCurrent >= mpAggregate->mpItems.size();}void * CConcreteIterator::CurrentItem() { int nSubscript = 0; for each(auto i in mpAggregate->mpItems) { if (nSubscript++ == mnCurrent) { return i; } } return NULL;}#pragma once#include "stdafx.h"#include "Iterator.h"#include#includeusing namespace std;int main() { CConcreteAggregate *pA = new CConcreteAggregate(); pA->Insert(new string("node-1")); pA->Insert(new string("node-2")); string * pStr = new string("node-3"); pA->Insert(pStr); Iterator *pIteratorA = new CConcreteIterator(pA); while (!pIteratorA->IsDone()) { cout << *((string*)pIteratorA->CurrentItem()) << endl; pIteratorA->Next(); } pA->Remove(pStr); Iterator *pIteratorB = pA->CreateIterator(); while (!pIteratorB->IsDone()) { cout << *((string*)pIteratorB->CurrentItem()) << endl; pIteratorB->Next(); } pA->Clear(); delete pIteratorA; delete pIteratorB; delete pA; delete pStr; getchar(); return 0;}
版权声明:本文内容由网络用户投稿,版权归原作者所有,本站不拥有其著作权,亦不承担相应法律责任。如果您发现本站中有涉嫌抄袭或描述失实的内容,请联系我们jiasou666@gmail.com 处理,核实后本网站将在24小时内删除侵权内容。
暂时没有评论,来抢沙发吧~