173. Binary Search Tree Iterator
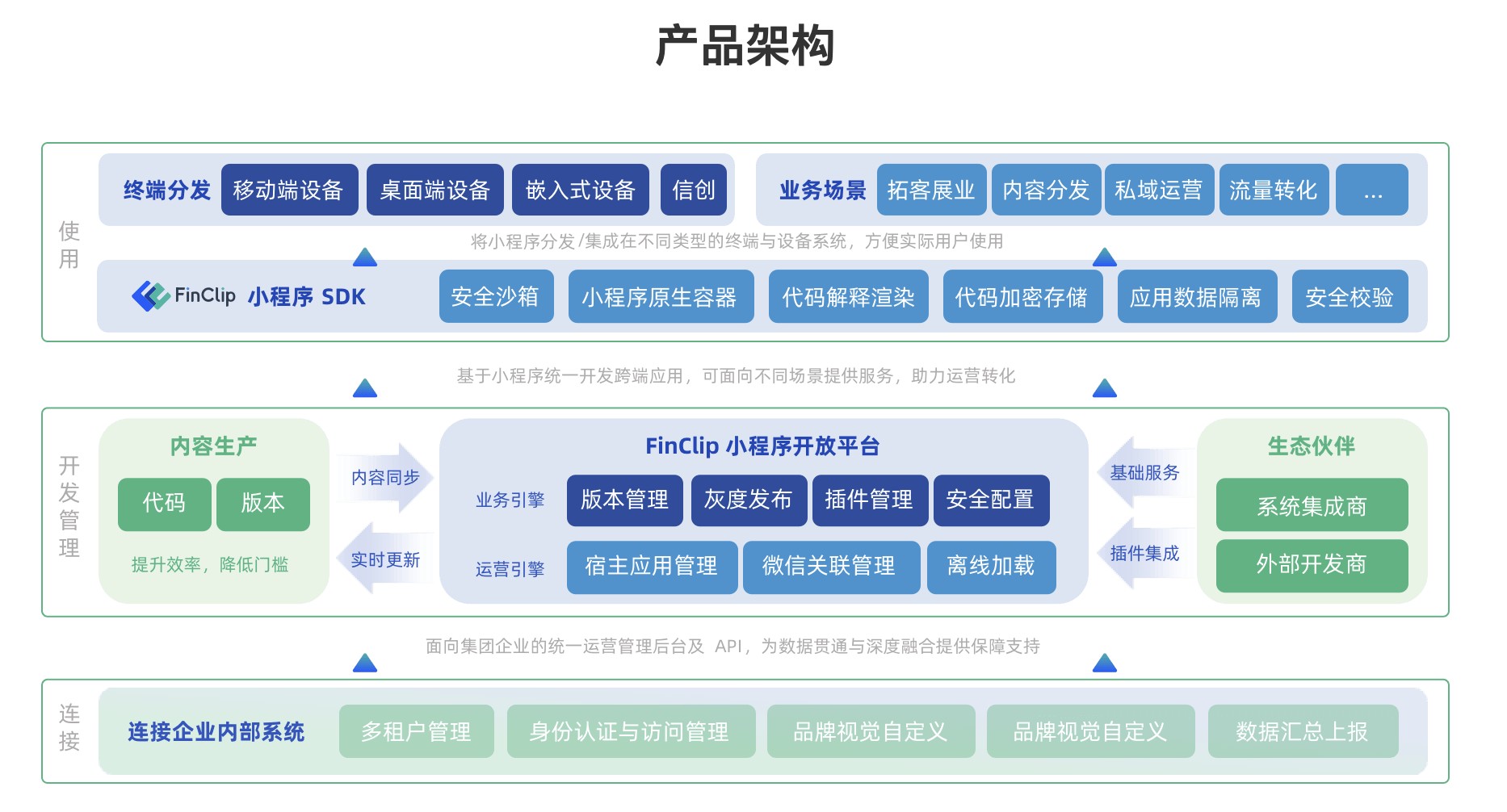
Implement an iterator over a binary search tree (BST). Your iterator will be initialized with the root node of a BST.
Calling next() will return the next smallest number in the BST.
Note: next() and hasNext() should run in average O(1) time and uses O(h) memory, where h is the height of the tree.
/** * Definition for binary tree * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode(int x) { val = x; } * } */public class BSTIterator { private Stack stack=new Stack(); public BSTIterator(TreeNode root) { if (root != null) pushLeft(root); } /** @return whether we have a next smallest number */ public boolean hasNext() { return !stack.isEmpty(); } /** @return the next smallest number */ public int next() { TreeNode top = stack.peek(); stack.pop(); if(top.right!=null) pushLeft(top.right); return top.val; } public void pushLeft(TreeNode root) { stack.push(root); TreeNode rootTemp = root.left; while (rootTemp != null) { stack.push(rootTemp); rootTemp = rootTemp.left; } }}/** * Your BSTIterator will be called like this: * BSTIterator i = new BSTIterator(root); * while (i.hasNext()) v[f()] = i.next(); */
/** * Definition for binary tree * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode(int x) { val = x; } * } */public class BSTIterator { ArrayList q; int index=0; public BSTIterator(TreeNode root) { q=new ArrayList<>(); if(root!=null) setVal(root); } void setVal(TreeNode root){ if(root.left!=null){ setVal(root.left); } q.add(root.val); if(root.right!=null){ setVal(root.right); } } /** @return the next smallest number */ public int next() { return q.get(index++); } /** @return whether we have a next smallest number */ public boolean hasNext() { return index/** * Definition for binary tree * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode(int x) { val = x; } * } */public class BSTIterator { ArrayList nodeSorted; int index; public BSTIterator(TreeNode root) { nodeSorted = new ArrayList<>(); index = -1; inorder(root); } private void inorder(TreeNode root){ if(root == null) return; inorder(root.left); nodeSorted.add(root.val); inorder(root.right); } /** @return the next smallest number */ public int next() { return nodeSorted.get(++index); } /** @return whether we have a next smallest number */ public boolean hasNext() { return index + 1 < nodeSorted.size(); }}/** * Your BSTIterator will be called like this: * BSTIterator i = new BSTIterator(root); * while (i.hasNext()) v[f()] = i.next(); */
版权声明:本文内容由网络用户投稿,版权归原作者所有,本站不拥有其著作权,亦不承担相应法律责任。如果您发现本站中有涉嫌抄袭或描述失实的内容,请联系我们jiasou666@gmail.com 处理,核实后本网站将在24小时内删除侵权内容。
暂时没有评论,来抢沙发吧~