Asp.NetCore 读取配置文件帮助类
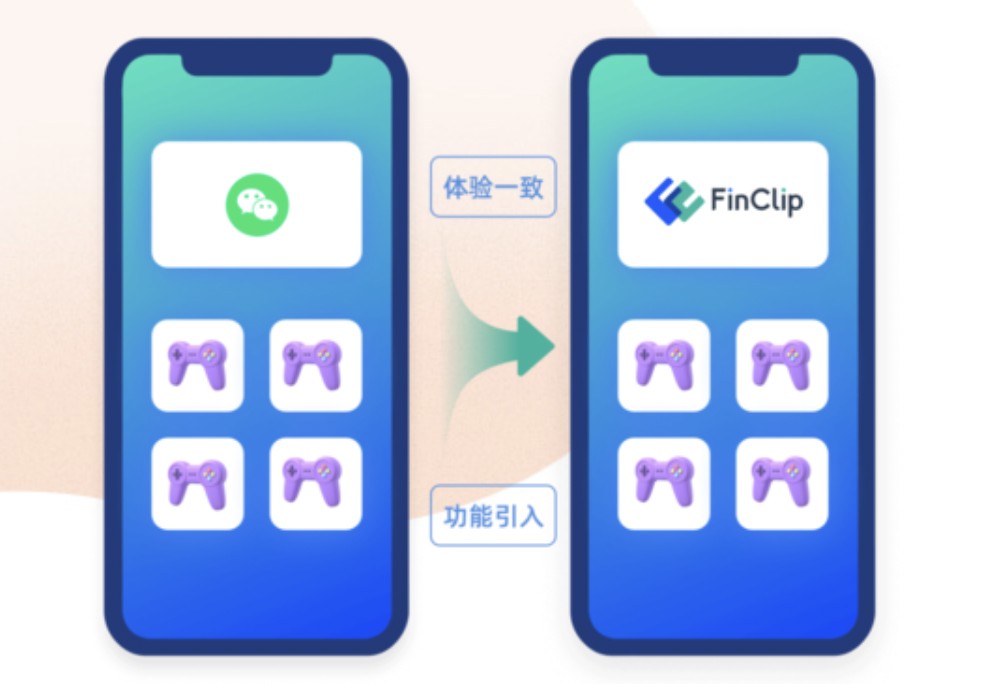
/// /// 读取配置文件信息 /// public class ConfigExtensions { public static IConfiguration Configuration { get; set; } static ConfigExtensions() { Configuration = new ConfigurationBuilder() .Add(new jsonConfigurationSource { Path = "appsettings.json", ReloadOnChange = true }) .Build(); } /// /// 获得配置文件的对象值 /// /// /// /// public static string GetJson(string jsonPath, string key) { if (string.IsNullOrEmpty(jsonPath) || string.IsNullOrEmpty(key)) return null; IConfiguration config = new ConfigurationBuilder().AddJsonFile(jsonPath).Build();//json文件地址 return config.GetSection(key).Value;//json某个对象 } /// /// 根据配置文件和Key获得对象 /// /// /// 文件名称 /// 节点Key /// public static T GetAppSettings(string fileName, string key) where T : class, new() { if (string.IsNullOrEmpty(fileName) || string.IsNullOrEmpty(key)) return null; var baseDir = AppContext.BaseDirectory + "json/"; var currentClassDir = baseDir; IConfiguration config = new ConfigurationBuilder() .SetBasePath(currentClassDir) .Add(new JsonConfigurationSource { Path = fileName, Optional = false, ReloadOnChange = true }).Build(); var appconfig = new ServiceCollection().AddOptions() .Configure(config.GetSection(key)) .BuildServiceProvider() .GetService>() .Value; return appconfig; } /// /// 获取自定义配置文件配置 /// /// 配置模型 /// 根节点 /// 配置文件名称 /// public T GetAppSettingss(string key, string configPath) where T : class, new() { if (string.IsNullOrEmpty(key) || string.IsNullOrEmpty(configPath)) return null; IConfiguration config = new ConfigurationBuilder().Add (new JsonConfigurationSource { Path = configPath, ReloadOnChange = true }).Build(); var appconfig = new ServiceCollection() .AddOptions() .Configure(config.GetSection(key)) .BuildServiceProvider() .GetService>() .Value; return appconfig; } /// /// 获取自定义配置文件配置(异步方式) /// /// 配置模型 /// 根节点 /// 配置文件名称 /// public async Task GetAppSettingsAsync(string key, string configPath) where T : class, new() { if (string.IsNullOrEmpty(key) || string.IsNullOrEmpty(configPath)) return null; IConfiguration config = new ConfigurationBuilder() .Add(new JsonConfigurationSource { Path = configPath, ReloadOnChange = true }).Build(); var appconfig = new ServiceCollection() .AddOptions() .Configure(config.GetSection(key)) .BuildServiceProvider() .GetService>() .Value; return await Task.Run(() => appconfig); } /// /// 获取自定义配置文件配置 /// /// 配置模型 /// 根节点 /// 配置文件名称 /// public List GetListAppSettings(string key, string configPath) where T : class, new() { if (string.IsNullOrEmpty(key) || string.IsNullOrEmpty(configPath)) return null; IConfiguration config = new ConfigurationBuilder() .Add(new JsonConfigurationSource { Path = configPath, ReloadOnChange = true }).Build(); var appconfig = new ServiceCollection() .AddOptions() .Configure>(config.GetSection(key)) .BuildServiceProvider() .GetService>>() .Value; return appconfig; } /// /// 获取自定义配置文件配置(异步方式) /// /// 配置模型 /// 根节点 /// 配置文件名称 /// public async Task> GetListAppSettingsAsync(string key, string configPath) where T : class, new() { if (string.IsNullOrEmpty(key) || string.IsNullOrEmpty(configPath)) return null; IConfiguration config = new ConfigurationBuilder() .Add(new JsonConfigurationSource { Path = configPath, ReloadOnChange = true }) .Build(); var appconfig = new ServiceCollection() .AddOptions() .Configure>(config.GetSection(key)) .BuildServiceProvider() .GetService>>() .Value; return await Task.Run(() => appconfig); } }
文件读取类:
public class FileHelperCore { private static IHostingEnvironment _hostingEnvironment = new HttpContextAccessor().HttpContext.RequestServices.GetService (typeof(IHostingEnvironment)) as IHostingEnvironment; /// /// 目录分隔符 /// windows "\" OSX and Linux "/" /// private static string DirectorySeparatorChar = Path.DirectorySeparatorChar.ToString(); /// /// 包含应用程序的目录的绝对路径 /// private static string _ContentRootPath = _hostingEnvironment.ContentRootPath; #region 检测指定路径是否存在 /// /// 检测指定路径是否存在 /// /// 路径 /// public static bool IsExist(string path) { if (string.IsNullOrEmpty(path)) return false; return IsDirectory(MapPath(path)) ? Directory.Exists(MapPath(path)) : File.Exists(MapPath(path)); } /// /// 检测指定路径是否存在(异步方式) /// /// 路径 /// public static async Task IsExistAsync(string path) { if (string.IsNullOrEmpty(path)) return false; return await Task.Run(() => IsDirectory(MapPath(path)) ? Directory.Exists(MapPath(path)) : File.Exists(MapPath(path))); } #endregion #region 检测目录是否为空 /// /// 检测目录是否为空 /// /// 路径 /// public static bool IsEmptyDirectory(string path) { if (string.IsNullOrEmpty(path)) return false; return Directory.GetFiles(MapPath(path)).Length <= 0 && Directory.GetDirectories(MapPath(path)).Length <= 0; } /// /// 检测目录是否为空 /// /// 路径 /// public static async Task IsEmptyDirectoryAsync(string path) { if (string.IsNullOrEmpty(path)) return false; return await Task.Run(() => Directory.GetFiles(MapPath(path)).Length <= 0 && Directory.GetDirectories(MapPath(path)).Length <= 0); } #endregion #region 创建目录 /// /// 创建目录 /// /// 路径 public static void CreateFiles(string path) { if (string.IsNullOrEmpty(path)) return; try { if (IsDirectory(MapPath(path))) Directory.CreateDirectory(MapPath(path)); else File.Create(MapPath(path)).Dispose(); } catch (Exception ex) { throw ex; } } #endregion #region 删除文件或目录 /// /// 删除目录或文件 /// /// 路径 public static void DeleteFiles(string path) { if (string.IsNullOrEmpty(path)) return; try { if (IsExist(path)) { if (IsDirectory(MapPath(path))) Directory.Delete(MapPath(path)); else File.Delete(MapPath(path)); } } catch (Exception ex) { throw ex; } } /// /// 清空目录下所有文件及子目录,依然保留该目录 /// /// public static void ClearDirectory(string path) { if (string.IsNullOrEmpty(path)) return; if (IsExist(path)) { //目录下所有文件 string[] files = Directory.GetFiles(MapPath(path)); foreach (var file in files) { DeleteFiles(file); } //目录下所有子目录 string[] directorys = Directory.GetDirectories(MapPath(path)); foreach (var dir in directorys) { DeleteFiles(dir); } } } #endregion #region 判断文件是否为隐藏文件(系统独占文件) /// /// 检测文件或文件夹是否为隐藏文件 /// /// 路径 /// public static bool IsHiddenFile(string path) { if (string.IsNullOrEmpty(path)) return false; return IsDirectory(MapPath(path)) ? InspectHiddenFile( new DirectoryInfo(MapPath(path))) : InspectHiddenFile(new FileInfo(MapPath(path))); } /// /// 检测文件或文件夹是否为隐藏文件(异步方式) /// /// 路径 /// public static async Task IsHiddenFileAsync(string path) { if (string.IsNullOrEmpty(path)) return false; return await Task.Run(() => IsDirectory(MapPath(path)) ? InspectHiddenFile(new DirectoryInfo(MapPath(path))) : InspectHiddenFile(new FileInfo(MapPath(path)))); } /// /// 私有方法 文件是否为隐藏文件(系统独占文件) /// /// /// private static bool InspectHiddenFile(FileSystemInfo fileSystemInfo) { if (fileSystemInfo == null) return false; if (fileSystemInfo.Name.StartsWith(".")) { return true; } else if (fileSystemInfo.Exists && ((fileSystemInfo.Attributes & FileAttributes.Hidden) != 0 || (fileSystemInfo.Attributes & FileAttributes.System) != 0)) { return true; } return false; } #endregion #region 文件操作 #region 复制文件 /// /// 复制文件内容到目标文件夹 /// /// 源文件 /// 目标文件夹 /// 是否可以覆盖 public static void Copy(string sourcePath, string targetPath, bool isOverWrite = true) { if (string.IsNullOrEmpty(sourcePath) || string.IsNullOrEmpty(targetPath)) return; File.Copy(MapPath(sourcePath), MapPath(targetPath) + GetFileName(sourcePath), isOverWrite); } /// /// 复制文件内容到目标文件夹 /// /// 源文件 /// 目标文件夹 /// 新文件名称 /// 是否可以覆盖 public static void Copy(string sourcePath, string targetPath, string newName, bool isOverWrite = true) { if (string.IsNullOrEmpty(sourcePath) || string.IsNullOrEmpty(targetPath)) return; File.Copy(MapPath(sourcePath), MapPath(targetPath) + newName, isOverWrite); } #endregion #region 移动文件 /// /// 移动文件到目标目录 /// /// 源文件 /// 目标目录 public static void Move(string sourcePath, string targetPath) { if (string.IsNullOrEmpty(sourcePath) || string.IsNullOrEmpty(targetPath)) return; string sourceFileName = GetFileName(sourcePath); //如果目标目录不存在则创建 if (IsExist(targetPath)) { CreateFiles(targetPath); } else { //如果目标目录存在同名文件则删除 if (IsExist(Path.Combine(MapPath(targetPath), sourceFileName))) { DeleteFiles(Path.Combine(MapPath(targetPath), sourceFileName)); } } File.Move(MapPath(sourcePath), Path.Combine(MapPath(targetPath), sourcePath)); } #endregion /// /// 获取文件名和扩展名 /// /// 文件路径 /// public static string GetFileName(string path) { if (string.IsNullOrEmpty(path)) return path; return Path.GetFileName(MapPath(path)); } /// /// 获取文件名不带扩展名 /// /// 文件路径 /// public static string GetFileNameWithOutExtension(string path) { if (string.IsNullOrEmpty(path)) return path; return Path.GetFileNameWithoutExtension(MapPath(path)); } /// /// 获取文件扩展名 /// /// 文件路径 /// public static string GetFileExtension(string path) { if (string.IsNullOrEmpty(path)) return path; return Path.GetExtension(MapPath(path)); } #endregion #region 获取文件绝对路径 /// /// 获取文件绝对路径 /// /// 文件路径 /// public static string MapPath(string path) { if (string.IsNullOrEmpty(path)) return path; return Path.Combine(_ContentRootPath, path.TrimStart('~', '/').Replace("/", DirectorySeparatorChar)); } /// /// 获取文件绝对路径(异步方式) /// /// 文件路径 /// public static async Task MapPathAsync(string path) { if (string.IsNullOrEmpty(path)) return path; return await Task.Run(()=> Path.Combine(_ContentRootPath,path.TrimStart('~','/'). Replace("/", DirectorySeparatorChar))); } /// /// 是否为目录或文件夹 /// /// 路径 /// public static bool IsDirectory(string path) { if (string.IsNullOrEmpty(path)) return false; if (path.EndsWith(DirectorySeparatorChar)) return true; else return false; } #endregion #region 物理路径转虚拟路径 public static string PhysicalToVirtual(string physicalPath) { if (string.IsNullOrEmpty(physicalPath)) return physicalPath; return physicalPath.Replace(_ContentRootPath, "").Replace(DirectorySeparatorChar, "/"); } #endregion #region 文件格式 /// /// 是否可添加水印 /// /// 文件扩展名,不含“.” /// public static bool IsCanWater(string _fileExt) { if (string.IsNullOrEmpty(_fileExt)) return false; var images = new List { "jpg", "jpeg" }; if (images.Contains(_fileExt.ToLower())) return true; return false; } /// /// 是否为图片 /// /// 文件扩展名,不含“.” /// public static bool IsImage(string _fileExt) { if (string.IsNullOrEmpty(_fileExt)) return false; var images = new List { "bmp", "gif", "jpg", "jpeg", "png" }; if (images.Contains(_fileExt.ToLower())) return true; return false; } /// /// 是否为视频 /// /// 文件扩展名,不含“.” /// public static bool IsVideos(string _fileExt) { if (string.IsNullOrEmpty(_fileExt)) return false; var videos=new List { "rmvb", "mkv", "ts", "wma", "avi", "rm", "mp4", "flv", "mpeg", "mov", "3gp", "mpg" }; if (videos.Contains(_fileExt.ToLower())) return true; return false; } /// /// 是否为音频 /// /// 文件扩展名,不含“.” /// public static bool IsMusics(string _fileExt) { if (string.IsNullOrEmpty(_fileExt)) return false; var musics = new List { "mp3", "wav" }; if (musics.Contains(_fileExt.ToLower())) return true; return false; } /// /// 是否为文档 /// /// 文件扩展名,不含“.” /// public static bool IsDocument(string _fileExt) { if (string.IsNullOrEmpty(_fileExt)) return false; var documents = new List { "doc", "docx", "xls", "xlsx", "ppt", "pptx", "txt", "pdf" }; if (documents.Contains(_fileExt.ToLower())) return true; return false; } #endregion #region 文件图标 public static string FindFileIcon(string fileExt) { if (string.IsNullOrEmpty(fileExt)) return fileExt; if (IsImage(fileExt)) return "fa fa-image"; if (IsVideos(fileExt)) return "fa fa-film"; if (IsMusics(fileExt)) return "fa fa-music"; if(IsDocument(fileExt)) switch (fileExt.ToLower()) { case ".xls": case ".xlsx": return "fa fa-file-excel-o"; case ".ppt": case ".pptx": return "fa fa-file-powerpoint-o"; case ".pdf": return "fa fa-file-pdf-o"; case ".txt": return "fa fa-file-text-o"; default: return "fa fa-file-word-o"; } if (fileExt.ToLower() == "zip" || fileExt.ToLower() == "rar") return "fa fa-file-zip-o"; else return "fa fa-file"; } #endregion #region 文件大小转换 /// /// 文件大小转为B、KB、MB、GB... /// /// /// public static string FileSizeTransf(long size) { if (size==0) return null; String[] units = new String[] { "B", "KB", "MB", "GB", "TB", "PB" }; long mod = 1024; int i = 0; while (size > mod) { size /= mod; i++; } return size + units[i]; } #endregion #region 获取目录下所有文件 public static List FindFiles(string path, string staticFiles = "/ { if (string.IsNullOrEmpty(path)) return null; string[] folders = Directory.GetDirectories(MapPath(path), "*", SearchOption.AllDirectories); var Files = new List(); foreach (var folder in folders) { foreach (var fsi in new DirectoryInfo(folder).GetFiles()) { Files.Add(new FilesInfo() { Name = fsi.Name, FullName = fsi.FullName, FileExt = fsi.Extension, FileOriginalSize = fsi.Length, FileSize = FileSizeTransf(fsi.Length), FileIcon = FindFileIcon(fsi.Extension.Remove(0, 1)), FileName = PhysicalToVirtual(fsi.FullName).Replace(staticFiles, ""), FileStyle = IsImage(fsi.Extension.Remove(0, 1)) ? "images" : IsDocument(fsi.Extension.Remove(0, 1)) ? "documents" : IsVideos(fsi.Extension.Remove(0, 1)) ? "videos" : IsMusics(fsi.Extension.Remove(0, 1)) ? "musics" : "others", CreateDate = fsi.CreationTime, LastWriteDate = fsi.LastWriteTime, LastAccessDate = fsi.LastAccessTime }); } } return Files; } /// /// 获得指定文件夹下面的所有文件 /// /// /// /// public static List ResolveFileInfo(string path, string staticFiles = "/ { if (string.IsNullOrEmpty(path)) return null; var foldersPath = MapPath(path); var Files = new List(); foreach (var fsi in new DirectoryInfo(foldersPath).GetFiles()) { Files.Add(new FilesInfo() { Name = fsi.Name, FullName = fsi.FullName, FileExt = fsi.Extension, FileOriginalSize = fsi.Length, FileSize = FileSizeTransf(fsi.Length), FileIcon = FindFileIcon(fsi.Extension.Remove(0, 1)), FileName = PhysicalToVirtual(fsi.FullName).Replace(staticFiles, ""), FileStyle = IsImage(fsi.Extension.Remove(0, 1)) ? "images" : IsDocument(fsi.Extension.Remove(0, 1)) ? "documents" : IsVideos(fsi.Extension.Remove(0, 1)) ? "videos" : IsMusics(fsi.Extension.Remove(0, 1)) ? "musics" : "others", CreateDate = fsi.CreationTime, LastWriteDate = fsi.LastWriteTime, LastAccessDate = fsi.LastAccessTime }); } return Files; } #endregion } public class FilesInfo { /// /// 文件名称 /// public string Name { get; set; } /// /// 文件物理路径 /// public string FullName { get; set; } /// /// 扩展名 /// public string FileExt { get; set; } /// /// 原始大小(字节) /// public long FileOriginalSize { get; set; } /// /// 文件大小 /// public string FileSize { get; set; } /// /// 文件虚拟路径 /// public string FileName { get; set; } /// /// 文件类型 /// public string FileStyle { get; set; } /// /// 文件图标 /// public string FileIcon { get; set; } /// /// 创建时间 /// public DateTime CreateDate { get; set; } /// /// 最后修改时间 /// public DateTime LastWriteDate { get; set; } /// /// 最后访问时间 /// public DateTime LastAccessDate { get; set; } }
版权声明:本文内容由网络用户投稿,版权归原作者所有,本站不拥有其著作权,亦不承担相应法律责任。如果您发现本站中有涉嫌抄袭或描述失实的内容,请联系我们jiasou666@gmail.com 处理,核实后本网站将在24小时内删除侵权内容。
暂时没有评论,来抢沙发吧~