android算法实现房贷计算器
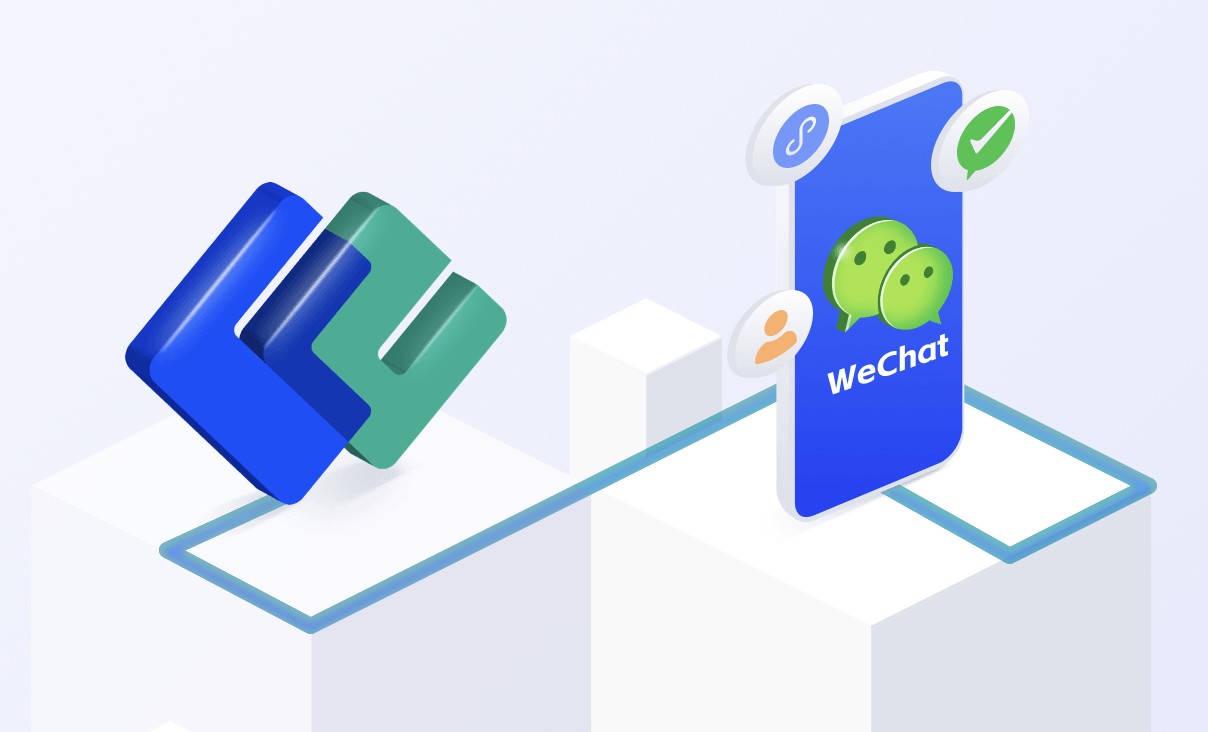
说明:最近碰到一个需求,用算法手写一个房贷计算器,包括等额本金和等额本息,花了一天实现了这个功能,源码全部贴出来了,计算公式也在代码里,需要请自取
step1:
package com.example.myapplication;
import android.view.View;
import androidx.recyclerview.widget.RecyclerView;
/**
* Created by kee on 2017/12/25.
*/
public abstract class BaseRecyclerViewAdapter extends RecyclerView.Adapter implements View.OnClickListener, View.OnLongClickListener {
private OnItemClickListener onItemClickListener;
private OnItemLongClickListener onItemLongClickListener;
@Override
public void onBindViewHolder(VH holder, int position) {
holder.itemView.setTag(position);
holder.itemView.setOnClickListener(this);
holder.itemView.setOnLongClickListener(this);
}
@Override
public void onClick(View v) {
if (onItemClickListener != null) {
onItemClickListener.onItemClick((Integer) v.getTag());
}
}
@Override
public boolean onLongClick(View v) {
if (onItemLongClickListener != null) {
return onItemLongClickListener.onLongClick((Integer) v.getTag());
}
return false;
}
public void setOnItemClickListener(OnItemClickListener onItemClickListener) {
this.onItemClickListener = onItemClickListener;
}
public void setOnItemLongClickListener(OnItemLongClickListener onItemLongClickListener) {
this.onItemLongClickListener = onItemLongClickListener;
}
public interface OnItemClickListener {
void onItemClick(int position);
}
public interface OnItemLongClickListener {
boolean onLongClick(int position);
}
}
step2:
package com.example.myapplication;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import java.util.List;
import androidx.recyclerview.widget.RecyclerView;
/**
* groups
* Created by kee on 2017/8/18.
*/
public class GroupInfoAdapter extends BaseRecyclerViewAdapter {
private Context mContext;
private List mGroups;
private boolean enable;
public GroupInfoAdapter(Context context, List groups) {
this.mContext = context;
this.mGroups = groups;
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View itemView = LayoutInflater.from(mContext).inflate(R.layout.item_device_group, parent, false);
ViewHolder holder = new ViewHolder(itemView);
holder.tv_id = (TextView) itemView.findViewById(R.id.tv_id);
holder.tv_all = (TextView) itemView.findViewById(R.id.tv_all);
holder.tv_year_factor = (TextView) itemView.findViewById(R.id.tv_year_factor);
holder.tv_month_factor = (TextView) itemView.findViewById(R.id.tv_month_factor);
holder.tv_year_int = (TextView) itemView.findViewById(R.id.tv_year_int);
holder.tv_month_int = (TextView) itemView.findViewById(R.id.tv_month_int);
holder.tv_li_month = (TextView) itemView.findViewById(R.id.tv_li_month);
holder.tv_ben_month = (TextView) itemView.findViewById(R.id.tv_ben_month);
holder.tv_all_month = (TextView) itemView.findViewById(R.id.tv_all_month);
holder.tv_java_money = (TextView) itemView.findViewById(R.id.tv_java_money);
holder.tv_remain_money = (TextView) itemView.findViewById(R.id.tv_remain_money);
holder.tv_time = (TextView) itemView.findViewById(R.id.tv_time);
holder.tv_all_money = (TextView) itemView.findViewById(R.id.tv_all_money);
return holder;
}
@Override
public int getItemCount() {
return mGroups == null ? 0 : mGroups.size();
}
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
super.onBindViewHolder(holder, position);
LoanBean group = mGroups.get(position);
holder.tv_id.setText(String.valueOf(group.getId()));
holder.tv_all.setText(String.format("%.2f", group.getAll()));
holder.tv_year_factor.setText(String.format("%.3f", group.getYearFactor()));
holder.tv_month_factor.setText(String.format("%.3f", group.getMonthFactor()));
holder.tv_year_int.setText(String.format("%.2f", group.getYearInt()));
holder.tv_month_int.setText(String.format("%.2f", group.getMonthInt()));
holder.tv_li_month.setText(String.format("%.2f", group.getLiMonth()));
holder.tv_ben_month.setText(String.format("%.2f", group.getBenMonth()));
holder.tv_all_month.setText(String.format("%.2f", group.getAllMonth()));
holder.tv_remain_money.setText(String.format("%.2f", group.getRemainMoney()));
holder.tv_java_money.setText(String.format("%.2f", group.getJavaMoney()));
holder.tv_time.setText(group.getTime(group.getYear(),group.getMonth(),group.getDay()));
holder.tv_all_money.setText(String.format("%.2f", group.getAllMoney()));
}
class ViewHolder extends RecyclerView.ViewHolder {
TextView tv_id, tv_all, tv_year_factor,
tv_month_factor, tv_year_int, tv_month_int,
tv_li_month, tv_ben_month, tv_all_month,
tv_remain_money, tv_java_money,tv_time,tv_all_money;
public ViewHolder(View itemView) {
super(itemView);
}
}
}
step3:
package com.example.myapplication;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.widget.NestedScrollView;
import androidx.fragment.app.FragmentActivity;
import androidx.recyclerview.widget.LinearLayoutManager;
import androidx.recyclerview.widget.RecyclerView;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
public class HelloActivity extends FragmentActivity {
double all = 0;
double yearFactor = 0;
double monthFactor = 0;
double yearInt = 0;
double monthInt = 0;
double liMonth = 0;
double benMonth = 0;
double allMonth = 0;
double remainMoney = 0;
double javaMoney = 0;
private HashMap map;
private GroupInfoAdapter mAdapter;
private RecyclerView rv_group;
private List list;
private EditText et_year_factor;
private EditText et_all;
private EditText et_year_int;
private Button btn_search, btn_search2;
private EditText et_year;
private EditText et_month;
private EditText et_day;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
map = new HashMap<>();
list = new ArrayList<>();
all = 1000000;
yearFactor = 0.06;
monthFactor = yearFactor / 12;
yearInt = 30;
monthInt = yearInt * 12;
liMonth = remainMoney * monthFactor;
benMonth = remainMoney / monthInt;
allMonth = liMonth + benMonth;
remainMoney = all - javaMoney;
for (int i = 0; i < monthInt; i++) {
map.put(i, new LoanBean(i, all, ((monthInt+1)*all*monthFactor)/2+all,yearFactor, monthFactor, yearInt, monthInt,
(all - ((remainMoney / monthInt) * (i + 1))) * monthFactor,
remainMoney / monthInt,
(all - ((remainMoney / monthInt) * (i + 1))) * monthFactor + remainMoney / monthInt,
all - ((remainMoney / monthInt) * (i + 1)),
(remainMoney / monthInt) * (i + 1),
(i + 8) / 12 + 2022,
(i + 8) % 12,
9));
}
System.out.println(map);
if (!list.isEmpty()) {
list.clear();
}
Iterator iter = map.keySet().iterator();
while (iter.hasNext()) {
list.add(map.get(iter.next()));
}
rv_group = findViewById(R.id.rv_group);
et_year_factor = findViewById(R.id.et_year_factor);
et_all = findViewById(R.id.et_all);
et_year_int = findViewById(R.id.rt_year_int);
btn_search = findViewById(R.id.btn_search);
btn_search2 = findViewById(R.id.btn_search2);
et_year = findViewById(R.id.et_year);
et_month = findViewById(R.id.et_month);
et_day = findViewById(R.id.et_day);
mAdapter = new GroupInfoAdapter(HelloActivity.this, list);
rv_group.setLayoutManager(new LinearLayoutManager(HelloActivity.this));
rv_group.setAdapter(mAdapter);
btn_search.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String etYearFactorString = et_year_factor.getText().toString();
String etAllString = et_all.getText().toString();
String etYearIntString = et_year_int.getText().toString();
String currentYear = et_year.getText().toString();
String currentMonth = et_month.getText().toString();
String currentDay = et_day.getText().toString();
all = Double.parseDouble(etAllString) * 10000;
yearFactor = Double.parseDouble(etYearFactorString) / 100;
monthFactor = yearFactor / 12;
yearInt = Double.parseDouble(etYearIntString);
monthInt = yearInt * 12;
liMonth = remainMoney * monthFactor;
benMonth = remainMoney / monthInt;
allMonth = liMonth + benMonth;
remainMoney = all - javaMoney;
for (int i = 0; i < monthInt; i++) {
map.put(i, new LoanBean(i, all, ((monthInt+1)*all*monthFactor)/2+all,yearFactor, monthFactor, yearInt,
monthInt, (all - ((remainMoney / monthInt) * (i + 1))) * monthFactor, remainMoney / monthInt,
(all - ((remainMoney / monthInt) * (i + 1))) * monthFactor + remainMoney / monthInt,
all - ((remainMoney / monthInt) * (i + 1)),
(remainMoney / monthInt) * (i + 1),
(i + Integer.parseInt(currentMonth)) / 12 + Integer.parseInt(currentYear),
(i + Integer.parseInt(currentMonth)) % 12,
Integer.parseInt(currentDay)));
}
System.out.println(map);
if (!list.isEmpty()) {
list.clear();
}
Iterator iter = map.keySet().iterator();
while (iter.hasNext()) {
list.add(map.get(iter.next()));
}
mAdapter.notifyDataSetChanged();
}
});
btn_search2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String etYearFactorString = et_year_factor.getText().toString();
String etAllString = et_all.getText().toString();
String etYearIntString = et_year_int.getText().toString();
String currentYear = et_year.getText().toString();
String currentMonth = et_month.getText().toString();
String currentDay = et_day.getText().toString();
all = Double.parseDouble(etAllString) * 10000;
yearFactor = Double.parseDouble(etYearFactorString) / 100;
monthFactor = yearFactor / 12;
yearInt = Double.parseDouble(etYearIntString);
monthInt = yearInt * 12;
liMonth = remainMoney * monthFactor;
benMonth = remainMoney / monthInt;
allMonth = liMonth + benMonth;
remainMoney = all - javaMoney;
for (int i = 0; i < monthInt; i++) {
map.put(i, new LoanBean(i, all,
(all * (yearFactor / 12) * Math.pow((yearFactor / 12 + 1), 360) / (Math.pow((yearFactor / 12 + 1), 360) - 1))*monthInt,
yearFactor, monthFactor, yearInt,
monthInt,
all * monthFactor,
all * monthFactor * Math.pow((monthFactor + 1), monthInt) / (Math.pow((monthFactor + 1), monthInt) - 1) - all * monthFactor,
all * monthFactor * Math.pow((monthFactor + 1), monthInt) / (Math.pow((monthFactor + 1), monthInt) - 1),
all - (all * monthFactor * Math.pow((monthFactor + 1), monthInt) / (Math.pow((monthFactor + 1), monthInt) - 1) - all * monthFactor) * (i + 1),
(all * monthFactor * Math.pow((monthFactor + 1), monthInt) / (Math.pow((monthFactor + 1), monthInt) - 1) - all * monthFactor) * (i + 1),
(i + Integer.parseInt(currentMonth)) / 12 + Integer.parseInt(currentYear),
(i + Integer.parseInt(currentMonth)) % 12,
Integer.parseInt(currentDay)));
}
System.out.println(map);
if (!list.isEmpty()) {
list.clear();
}
Iterator iter = map.keySet().iterator();
while (iter.hasNext()) {
list.add(map.get(iter.next()));
}
mAdapter.notifyDataSetChanged();
}
});
}
}
step4:
package com.example.myapplication;
public class LoanBean {
/*
id 当月月数 唯一 id
all 总金额
yearFactor 年利率
monthFactor 月利率
yearInt 贷款年限
monthInt 贷款总期数
liMonth 当月偿还利息
benMonth 当月偿还本金
allMonth 当月还款总额
remainMoney 剩余未偿还本金
javaMoney 已偿还本金
year 还款年份
month 还款月份
* */
private int id;
private double all;
private double allMoney;
private double yearFactor;
private double monthFactor;
private double yearInt;
private double monthInt;
private double liMonth;
private double benMonth;
private double allMonth;
private double remainMoney;
private double javaMoney;
private int year;
private int month;
private int day;
public LoanBean(int id, double all, double allMoney, double yearFactor, double monthFactor, double yearInt, double monthInt, double liMonth, double benMonth, double allMonth, double remainMoney, double javaMoney, int year, int month,int day) {
this.id = id;
this.all = all;
this.allMoney = allMoney;
this.yearFactor = yearFactor;
this.monthFactor = monthFactor;
this.yearInt = yearInt;
this.monthInt = monthInt;
this.liMonth = liMonth;
this.benMonth = benMonth;
this.allMonth = allMonth;
this.remainMoney = remainMoney;
this.javaMoney = javaMoney;
this.year = year;
this.month = month;
this.day = day;
}
public double getAllMoney() {
return allMoney;
}
public void setAllMoney(double allMoney) {
this.allMoney = allMoney;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public double getAll() {
return all;
}
public void setAll(double all) {
this.all = all;
}
public double getYearFactor() {
return yearFactor;
}
public void setYearFactor(double yearFactor) {
this.yearFactor = yearFactor;
}
public double getMonthFactor() {
return monthFactor;
}
public void setMonthFactor(double monthFactor) {
this.monthFactor = monthFactor;
}
public double getYearInt() {
return yearInt;
}
public void setYearInt(double yearInt) {
this.yearInt = yearInt;
}
public double getMonthInt() {
return monthInt;
}
public void setMonthInt(double monthInt) {
this.monthInt = monthInt;
}
public double getLiMonth() {
return liMonth;
}
public void setLiMonth(double liMonth) {
this.liMonth = liMonth;
}
public double getBenMonth() {
return benMonth;
}
public void setBenMonth(double benMonth) {
this.benMonth = benMonth;
}
public double getAllMonth() {
return allMonth;
}
public void setAllMonth(double allMonth) {
this.allMonth = allMonth;
}
public double getRemainMoney() {
return remainMoney;
}
public void setRemainMoney(double remainMoney) {
this.remainMoney = remainMoney;
}
public double getJavaMoney() {
return javaMoney;
}
public void setJavaMoney(double javaMoney) {
this.javaMoney = javaMoney;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public int getMonth() {
return month;
}
public void setMonth(int month) {
this.month = month;
}
public int getDay() {
return day;
}
public void setDay(int day) {
this.day = day;
}
public String getTime(int year, int month,int day) {
if (month==0){
month=12;
}
return year+"年"+month+"月"+day+"日";
}
}
step5:
step6:
end
版权声明:本文内容由网络用户投稿,版权归原作者所有,本站不拥有其著作权,亦不承担相应法律责任。如果您发现本站中有涉嫌抄袭或描述失实的内容,请联系我们jiasou666@gmail.com 处理,核实后本网站将在24小时内删除侵权内容。
暂时没有评论,来抢沙发吧~