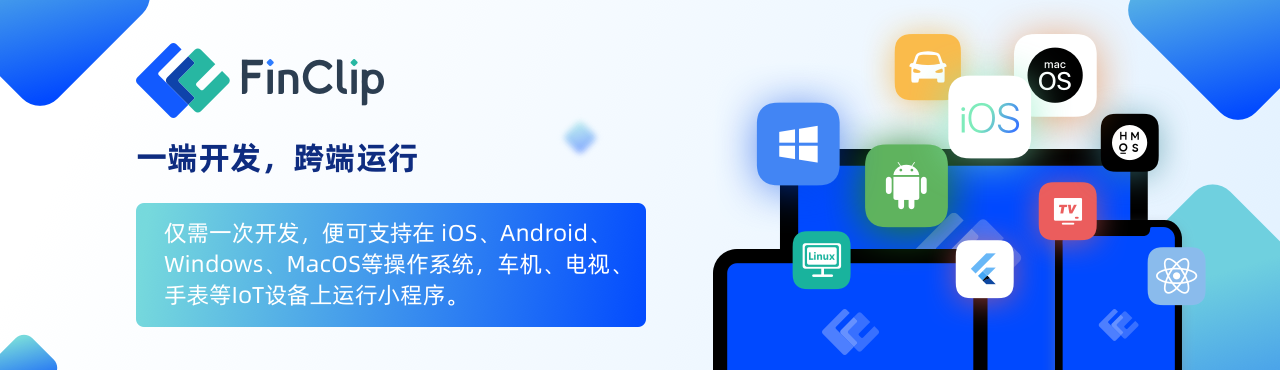
Fetch Inject 高性能网站的fetching异步加载程序和DOM注入顺序程序(fetch怎么读)
Fetch Inject 高性能网站的fetching异步加载程序和DOM注入顺序程序(fetch怎么读)
Fetch Inject
A fetching async loader and DOM injection sequencer for high-performance websites.
Background
This library implements Fetch Injection, a performance optimization technique for managing async script dependencies. Use Fetch Inject to dynamically import scripts in parallel (even across the network), and inject them into a document in a desired sequence. It also supports inlining of CSS, and can be extended to support other MIME types as well.
Waterfalls
Here are some example waterfalls using Fetch Inject.
Loading Bootstrap 4:
Loading jQuery, Transit, Hover Intent and Superfish:
Loading and initializing PhotoSwipe:
Playground
Test out the library using the latest version available on CDN using this Pen: https://codepen.io/jhabdas/pen/MpVeOE?editors=0012. Reference the Use Cases to get a feel for what it can do.
Installing
Fetch Inject is available on NPM, Bower and CDN. It ships in the following flavors: IIFE, UMD and ES6.
Get it on NPM with npm i fetch-injectBower with bower install fetch-injectCDN using jsDelivr
To download the lastest minified UMD bundle from the command line:
curl -L -o fetch-inject.umd.min.js https://go.habd.as/fetch-inject-umd-min
See the Development section for asset pipelines requiring vanilla AMD and CJS modules.
Syntax
Promise
Parameters
inputs Required. This defines the resources you wish to fetch. It must be an Array containing elements of type USVString or Request. promise Optional. A Promise to await before injecting fetched resources.
Return value
A Promise that resolves to an Array of Objects. Each Object contains a list of resolved properties of the Response Body used in the module, e.g.
[{ blob: { size: 44735, type: "application/javascript" }, text: "/*!↵ * Bootstrap v4.0.0-alpha.5 ... */"}, { blob: { size: 31000, type: "text/css" }, text: "/*!↵ * Font Awesome 4.7.0 ... */"}]
Use Cases
Preventing Script Blocking
Problem: External scripts can lead to jank or SPOF if not handled correctly.
Solution: Load external scripts without blocking:
fetchInject([ 'https://cdn.jsdelivr-/popper.js/1.0.0-beta.3/popper.min.js'])
This is a simple case to get you started. Don't worry, it gets better.
Loading Non-critical CSS
Problem: PageSpeed Insights and Lighthouse ding you for loading unnecessary styles on initial render.
Solution: Inline your critical CSS and load non-critical styles asynchronously:
Unlike loadCSS, Fetch Inject is smaller, doesn't use callbacks and ships a minifed UMD build for interop with CommonJS.
Lazyloading Scripts
Problem: You want to load a script in response to a user interaction without affecting your page load times.
Solution: Create an event listener, respond to the event and then destroy the listener.
const el = document.querySelector('details summary')el.onclick = (evt) => { fetchInject([ 'https://cdn.jsdelivr-/smooth-scroll/10.2.1/smooth-scroll.min.js' ]) el.onclick = null }
Here we are loading the smooth scroll polyfill when a user opens a details element, useful for displaying a collapsed and keyboard-friendly table of contents.
Responding to Asynchronous Scripts
Problem: You need to perform a synchronous operation immediately after an asynchronous script is loaded.
Solution: You could create a script element and use the async and onload attributes. Or you could...
fetchInject([ 'https://cdn.jsdelivr-/momentjs/2.17.1/moment.min.js']).then(() => { console.log(`Finish in less than ${moment().endOf('year').fromNow(true)}`)})
Ordering Script Dependencies
Problem: You have several scripts that depend on one another and you want to load them all asynchronously, in parallel, without causing race conditions.
Solution: Pass fetchInject to itself as a second argument, forming a promise recursion:
fetchInject([ 'https://npmcdn.com/bootstrap@4.0.0-alpha.5/dist/js/bootstrap.min.js'], fetchInject([ 'https://cdn.jsdelivr-/jquery/3.1.1/jquery.slim.min.js', 'https://npmcdn.com/tether@1.2.4/dist/js/tether.min.js']))
Managing Asynchronous Dependencies
Problem: You want to load some dependencies which require some dependencies, which require some dependencies. You want it all in parallel, and you want it now.
Solution: You could scatter some links into your document head, blocking initial page render, bloat your application bundle with scripts the user might not actually need. Or you could...
const tether = ['https://cdn.jsdelivr-/tether/1.4.0/tether.min.js']const drop = ['https://cdn.jsdelivr-/drop/1.4.2/js/drop.min.js']const tooltip = [ 'https://cdn.jsdelivr-/tooltip/1.2.0/tooltip.min.js', 'https://cdn.jsdelivr-/tooltip/1.2.0/tooltip-theme-arrows.css']fetchInject(tooltip, fetchInject(drop, fetchInject(tether))) .then(() => { new Tooltip({ target: document.querySelector('h1'), content: "You moused over the first H1!", classes: 'tooltip-theme-arrows', position: 'bottom center' }) })
What about jQuery dropdown menus? Sure why not...
fetchInject([ '/assets/js/main.js'], fetchInject([ '/assets/js/vendor/superfish.min.js'], fetchInject([ '/assets/js/vendor/jquery.transit.min.js', '/assets/js/vendor/jquery.hoverIntent.js'], fetchInject([ '/assets/js/vendor/jquery.min.js']))))
Loading and Handling Composite Libraries
Problem: You want to deep link to gallery images using PhotoSwipe without slowing down your page.
Solution: Download everything in parallel and instantiate when finished:
const container = document.querySelector('.pswp')const items = JSON.parse({{ .Params.gallery.main | jsonify }})fetchInject([ '/css/photoswipe.css', '/css/default-skin/default-skin.css', '/js/photoswipe.min.js', '/js/photoswipe-ui-default.min.js']).then(() => { const gallery = new PhotoSwipe(container, PhotoSwipeUI_Default, items) gallery.init()})
This example turns TOML into JSON, parses the object, downloads all of the PhotoSwipe goodies and then activates the PhotoSwipe gallery immediately when the interface is ready to be displayed.
Supported Browsers
All browsers with support for Fetch and Promises.
Fetch will become available in Safari in the Safari 10.1 release that ships with macOS Sierra 10.12.4 and Safari on iOS 10.3. Jon Davis, Web Technologies Evangelist
Progressive Enhancement
You don't need to polyfill fetch for older browsers when they already know how to load external scripts. Give them a satisfactory fallback experience instead.
In your document head get the async loading started right away if the browser supports it:
(function () { if (!(window.fetch && window.Promise)) return; fetchInject([ '/js/bootstrap.min.js' ], fetchInject([ '/js/jquery.slim.min.js', '/js/tether.min.js' ]))})()
Then, before the close of the document body, provide the traditional experience to avoid blocking the parser until content is visible:
(function () { if (!!(window.fetch && window.Promise)) return; document.write('