150. Evaluate Reverse Polish Notation
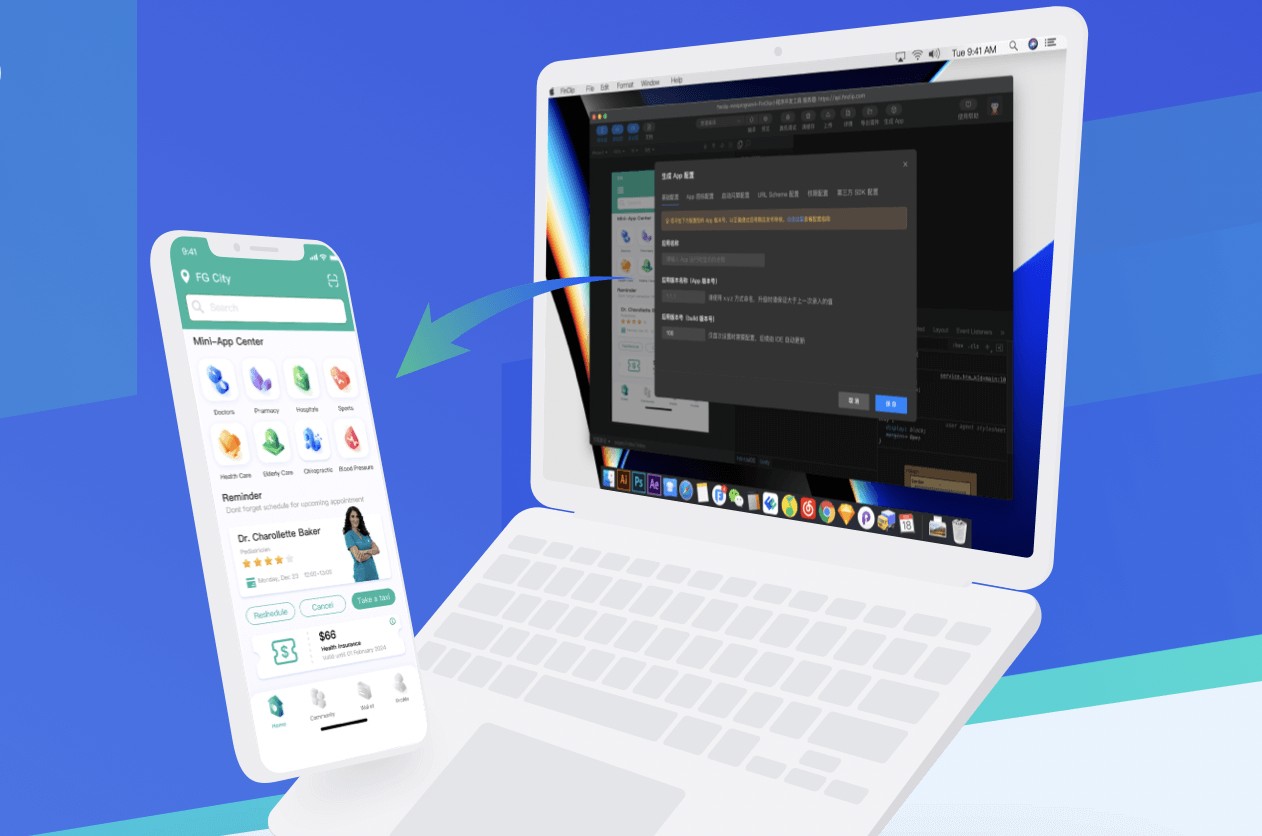
Evaluate the value of an arithmetic expression in Reverse Polish Notation.
Valid operators are +, -, *, /. Each operand may be an integer or another expression.
Some examples:
["2", "1", "+", "3", "*"] -> ((2 + 1) * 3) -> 9 ["4", "13", "5", "/", "+"] -> (4 + (13 / 5)) -> 6
class Solution { public int evalRPN(String[] tokens) { Stack s = new Stack(); String operators = "+-*/"; for(String token : tokens){ if(!operators.contains(token)){ s.push(Integer.valueOf(token)); continue; } int a = s.pop(); int b = s.pop(); if(token.equals("+")) { s.push(b + a); } else if(token.equals("-")) { s.push(b - a); } else if(token.equals("*")) { s.push(b * a); } else { s.push(b / a); } } return s.pop(); }}
class Solution { public int evalRPN(String[] tokens) { Stack stack = new Stack<>(); for (int i = 0; i < tokens.length; i++) { String str = tokens[i]; if (str.equals("+") || str.equals("*") || str.equals("/") || str.equals("-")) { int a2 = stack.pop(); int a1 = stack.pop(); if (str.equals("+")) stack.push(a1 + a2); else if (str.equals("-")) stack.push(a1 - a2); else if (str.equals("*")) stack.push(a1 * a2); else if (str.equals("/")) stack.push(a1 / a2); } else stack.push(Integer.parseInt(str)); } return stack.pop(); }}
class Solution { public int evalRPN(String[] tokens) { Deque intermediateResults = new LinkedList<>(); for(String s : tokens){ if("+-*/".contains(s)){ int y = intermediateResults.removeLast(); int x = intermediateResults.removeLast(); switch(s){ case "+": intermediateResults.add(x + y); break; case "-": intermediateResults.add(x - y); break; case "*": intermediateResults.add(x * y); break; case "/": intermediateResults.add(x / y); break; } } else { intermediateResults.add(Integer.parseInt(s)); } } return intermediateResults.poll(); }}
版权声明:本文内容由网络用户投稿,版权归原作者所有,本站不拥有其著作权,亦不承担相应法律责任。如果您发现本站中有涉嫌抄袭或描述失实的内容,请联系我们jiasou666@gmail.com 处理,核实后本网站将在24小时内删除侵权内容。
暂时没有评论,来抢沙发吧~