【VTK】create spline points
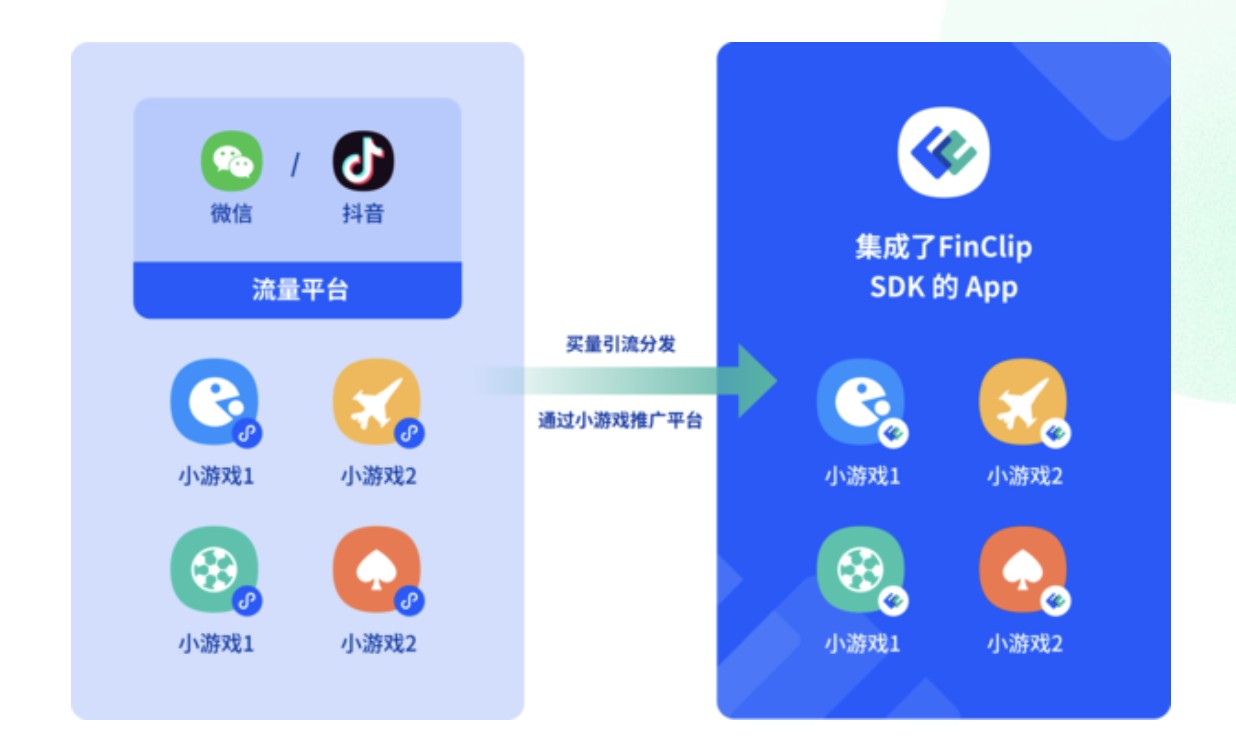
定义5个点
double p[5][3] = { {0.0, 4.0, 0.0}, {2.0, 0.0, 0.0}, {4.0, 2.0, 0.0}, {6.0, 0.0, 0.0}, {8.0, 4.0, 0.0} };
定义500个插值点
示例代码:
double p[5][3] = { {0.0, 4.0, 0.0}, {2.0, 0.0, 0.0}, {4.0, 2.0, 0.0}, {6.0, 0.0, 0.0}, {8.0, 4.0, 0.0} }; vtkSmartPointer points = vtkSmartPointer::New(); for( int i = 0; i < 5; ++i ) { points->InsertNextPoint( p[i] ); } vtkSmartPointer spline = vtkSmartPointer::New(); spline->SetPoints(points); vtkSmartPointer betaPoints = vtkSmartPointer::New(); int index = 0; int countOfDeltaPoints = 500; double step = 1.0 / ( countOfDeltaPoints - 1 ); for( double i = 0; i <= 1; i = i + step ) { double tmp[3] = { i, 0, 0 }; spline->Evaluate( tmp, tmp, NULL ); betaPoints->InsertPoint( index++, tmp ); }
使用vtkParametricFunctionSource的polyData,不用开发者自己来create points:
示例代码:
#include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include using namespace std;int main(int, char *[]){ vtkSmartPointer colors = vtkSmartPointer::New(); double p[5][3] = { {0.0, 4.0, 0.0}, {2.0, 0.0, 0.0}, {4.0, 2.0, 0.0}, {6.0, 0.0, 0.0}, {8.0, 4.0, 0.0} }; vtkSmartPointer points = vtkSmartPointer::New(); for( int i = 0; i < 5; ++i ) { points->InsertNextPoint( p[i] ); } vtkSmartPointer spline = vtkSmartPointer::New(); spline->SetPoints(points);/* * we can use this way to create some delta points on line. * vtkSmartPointer betaPoints = vtkSmartPointer::New(); int index = 0; int countOfDeltaPoints = 500; double step = 1.0 / ( countOfDeltaPoints - 1 ); for( double i = 0; i <= 1; i = i + step ) { double tmp[3] = { i, 0, 0 }; spline->Evaluate( tmp, tmp, NULL ); betaPoints->InsertPoint( index++, tmp ); } spline->SetPoints( betaPoints );*/ vtkSmartPointer functionSource = vtkSmartPointer::New(); functionSource->SetParametricFunction(spline); functionSource->Update(); vtkPolyData* polyData = functionSource->GetOutput(); vtkPoints* splinePoints = polyData->GetPoints(); // Setup actor and mapper vtkSmartPointer mapper = vtkSmartPointer::New(); mapper->SetInputConnection(functionSource->GetOutputPort()); vtkSmartPointer actor = vtkSmartPointer::New(); actor->SetMapper(mapper); actor->GetProperty()->SetColor( 1, 0, 0 ); actor->GetProperty()->SetLineWidth(3.0); // Setup render window, renderer, and interactor vtkSmartPointer renderer = vtkSmartPointer::New(); vtkSmartPointer renderWindow = vtkSmartPointer::New(); renderWindow->AddRenderer(renderer); vtkSmartPointer renderWindowInteractor = vtkSmartPointer::New(); renderWindowInteractor->SetRenderWindow(renderWindow); renderer->AddActor(actor); printf( "the count of spline points is %d\n", splinePoints->GetNumberOfPoints() ); for( int i = 0; i < splinePoints->GetNumberOfPoints(); ++i ) { vtkSmartPointer sphere = vtkSmartPointer::New(); sphere->SetRadius( 0.1 ); vtkSmartPointer sphereMapper = vtkSmartPointer::New(); sphereMapper->SetInputConnection( sphere->GetOutputPort() ); vtkSmartPointer sphereActor = vtkSmartPointer::New(); sphereActor->SetMapper( sphereMapper ); sphereActor->GetProperty()->SetColor( 0, 0, 1 ); sphereActor->VisibilityOn(); sphereActor->SetPosition( splinePoints->GetPoint(i) ); renderer->AddActor( sphereActor ); // text 2D vtkSmartPointer text2D = vtkSmartPointer::New(); text2D->SetText( QString::number( i+1 ).toStdString().c_str() ); vtkSmartPointer text2DTransform = vtkSmartPointer::New(); double *center = sphereActor->GetCenter(); text2DTransform->Translate( center[0], center[1] + 0.1, center[2] ); text2DTransform->Scale( 0.01, 0.01, 0.01 ); vtkSmartPointer text2DDataFilter = vtkSmartPointer::New(); text2DDataFilter->SetTransform( text2DTransform ); text2DDataFilter->SetInputConnection( text2D->GetOutputPort() ); vtkSmartPointer coords = vtkSmartPointer::New(); coords->SetCoordinateSystemToWorld(); vtkSmartPointer text2DMapper = vtkSmartPointer::New(); text2DMapper->SetInputConnection( text2DDataFilter->GetOutputPort() ); text2DMapper->SetTransformCoordinate( coords ); vtkSmartPointer text2DActor = vtkSmartPointer::New(); text2DActor->SetMapper( text2DMapper ); renderer->AddActor( text2DActor ); } renderer->SetBackground(colors->GetColor3d("Silver").GetData()); renderWindow->Render(); renderWindowInteractor->Start(); return EXIT_SUCCESS;}
现在,再创建一条线,使得两条线封闭。 接着,尝试将这些points作为平面的边线点,创造一个cell将points串起来,画出一个面。 这样沿着曲线画平面有一个问题: 曲面并不会100%的沿着边界点生成。 如下图:
实现代码:
#include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include using namespace std;int main(int, char *[]){ setbuf( stdout, NULL ); vtkSmartPointer colors = vtkSmartPointer::New(); double p[5][3] = { {0.0, 4.0, 0.0}, {2.0, 0.0, 0.0}, {4.0, 2.0, 0.0}, {6.0, 0.0, 0.0}, {8.0, 4.0, 0.0} }; vtkSmartPointer points = vtkSmartPointer::New(); for( int i = 0; i < 5; ++i ) { points->InsertNextPoint( p[i] ); } vtkSmartPointer spline = vtkSmartPointer::New(); spline->SetPoints(points); double p2[5][3] = { {0.0, 4.0, 0.0}, {2.0, 4.0, 0.0}, {4.0, 4.0, 0.0}, {6.0, 4.0, 0.0}, {8.0, 4.0, 0.0} }; for( int i = 0; i < 5; ++i ) { points->InsertNextPoint( p2[4 - i] ); }/* * we can use this way to create some delta points on line. * vtkSmartPointer betaPoints = vtkSmartPointer::New(); int index = 0; int countOfDeltaPoints = 500; double step = 1.0 / ( countOfDeltaPoints - 1 ); for( double i = 0; i <= 1; i = i + step ) { double tmp[3] = { i, 0, 0 }; spline->Evaluate( tmp, tmp, NULL ); betaPoints->InsertPoint( index++, tmp ); } spline->SetPoints( betaPoints );*/ vtkSmartPointer functionSource = vtkSmartPointer::New(); functionSource->SetParametricFunction(spline); functionSource->SetUResolution( 60 ); // generate 61 points. functionSource->GenerateNormalsOn(); functionSource->Update(); vtkPolyData* polyData = functionSource->GetOutput(); vtkPoints* splinePoints = polyData->GetPoints(); // Setup actor and mapper vtkSmartPointer mapper = vtkSmartPointer::New(); mapper->SetInputConnection(functionSource->GetOutputPort()); vtkSmartPointer actor = vtkSmartPointer::New(); actor->SetMapper(mapper); actor->GetProperty()->SetColor( 1, 0, 0 ); actor->GetProperty()->SetLineWidth(3.0); // Setup render window, renderer, and interactor vtkSmartPointer renderer = vtkSmartPointer::New(); vtkSmartPointer renderWindow = vtkSmartPointer::New(); renderWindow->AddRenderer(renderer); vtkSmartPointer renderWindowInteractor = vtkSmartPointer::New(); renderWindowInteractor->SetRenderWindow(renderWindow); renderer->AddActor( actor ); printf( "the count of spline points is %d\n", splinePoints->GetNumberOfPoints() ); for( int i = 0; i < splinePoints->GetNumberOfPoints(); ++i ) { vtkSmartPointer sphere = vtkSmartPointer::New(); sphere->SetRadius( 0.1 ); vtkSmartPointer sphereMapper = vtkSmartPointer::New(); sphereMapper->SetInputConnection( sphere->GetOutputPort() ); vtkSmartPointer sphereActor = vtkSmartPointer::New(); sphereActor->SetMapper( sphereMapper ); sphereActor->GetProperty()->SetColor( 0, 0, 1 ); sphereActor->VisibilityOn(); sphereActor->SetPosition( splinePoints->GetPoint(i) ); renderer->AddActor( sphereActor ); // text 2D vtkSmartPointer text2D = vtkSmartPointer::New(); text2D->SetText( QString::number( i+1 ).toStdString().c_str() ); vtkSmartPointer text2DTransform = vtkSmartPointer::New(); double *center = sphereActor->GetCenter(); text2DTransform->Translate( center[0], center[1] + 0.1, center[2] ); text2DTransform->Scale( 0.01, 0.01, 0.01 ); vtkSmartPointer text2DDataFilter = vtkSmartPointer::New(); text2DDataFilter->SetTransform( text2DTransform ); text2DDataFilter->SetInputConnection( text2D->GetOutputPort() ); vtkSmartPointer coords = vtkSmartPointer::New(); coords->SetCoordinateSystemToWorld(); vtkSmartPointer text2DMapper = vtkSmartPointer::New(); text2DMapper->SetInputConnection( text2DDataFilter->GetOutputPort() ); text2DMapper->SetTransformCoordinate( coords ); vtkSmartPointer text2DActor = vtkSmartPointer::New(); text2DActor->SetMapper( text2DMapper ); renderer->AddActor( text2DActor ); } // create cell to form plane vtkSmartPointer poly = vtkSmartPointer::New(); const int length = splinePoints->GetNumberOfPoints(); vtkIdType pts[ length ]; for( int i = 0; i < length; ++i ) { pts[i] = i; } poly->InsertNextCell( length, pts ); vtkSmartPointer planePd = vtkSmartPointer::New(); planePd->SetPoints( splinePoints ); planePd->SetPolys( poly ); vtkSmartPointer planeMapper = vtkSmartPointer::New(); planeMapper->SetInputData( planePd ); vtkSmartPointer planeActor = vtkSmartPointer::New(); planeActor->SetMapper( planeMapper ); renderer->AddActor( planeActor ); renderer->SetBackground(colors->GetColor3d("Silver").GetData()); renderWindow->Render(); renderWindowInteractor->Start(); return EXIT_SUCCESS;}
想要精确地画出曲面,可以使用微积分模型,利用这些边界点画出一个个小的四边形作为不同的cell,然后将这些不同的cell插入到vtkCellArray中。最后再构造vtkPolyData,mapper,actor等。
版权声明:本文内容由网络用户投稿,版权归原作者所有,本站不拥有其著作权,亦不承担相应法律责任。如果您发现本站中有涉嫌抄袭或描述失实的内容,请联系我们jiasou666@gmail.com 处理,核实后本网站将在24小时内删除侵权内容。
暂时没有评论,来抢沙发吧~