Windows下获取扬声器的一些信息
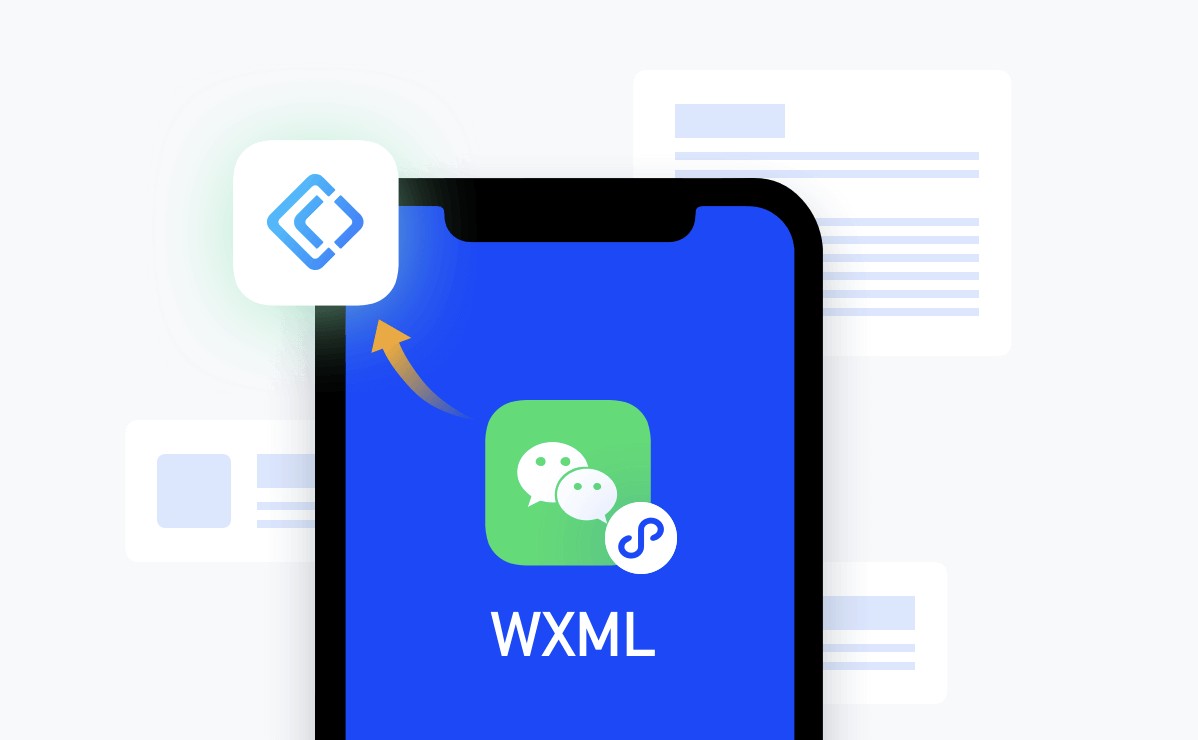
一、获取扬声器是否为静音和音量
#include "audio_utils.h"
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
// 获取默认设备的音量
float GetDefaultAudioDeviceVolume() {
CoInitializeEx(NULL, COINIT_MULTITHREADED);
// 创建com对象,创建IMMDeviceEnumerator对象
Microsoft::WRL::ComPtr enumerator = nullptr;
Microsoft::WRL::ComPtr device = nullptr;
Microsoft::WRL::ComPtr endpoint_volume = nullptr;
std::shared_ptr raii_ptr(nullptr, [&](void*) {
endpoint_volume = nullptr;
device = nullptr;
enumerator = nullptr;
CoUninitialize();
});
HRESULT hr = CoCreateInstance(__uuidof(MMDeviceEnumerator), NULL, CLSCTX_ALL,
__uuidof(IMMDeviceEnumerator), (void**)enumerator.GetAddressOf());
if (hr != S_OK) {
std::cout << "CoCreateInstance error" << std::endl;
return -1;
}
// 获取指定EDataFlow和ERole方向上的设备
// eRender:渲染设备的数据流方向,扬声器,对应eCapture:麦克风
// eConsole:游戏,系统通知声音和命令
hr = enumerator->GetDefaultAudioEndpoint(eRender, eConsole, device.GetAddressOf());
if (hr != S_OK) {
std::cout << "GetDefaultAudioEndpoint error" << std::endl;
return -1;
}
// 创建IAudioEndpointVolume对象
hr = device->Activate(__uuidof(IAudioEndpointVolume), CLSCTX_INPROC_SERVER,
NULL, (void**)endpoint_volume.GetAddressOf());
if (hr != S_OK) {
std::cout << "Activate error" << std::endl;
return -1;
}
float volume_sacle;
endpoint_volume->GetMasterVolumeLevelScalar(&volume_sacle);
return volume_sacle;
}
// 设置默认设备的音量
void SetDefaultAudioDeviceVolume(float volume) {
CoInitializeEx(NULL, COINIT_MULTITHREADED);
Microsoft::WRL::ComPtr enumerator = nullptr;
Microsoft::WRL::ComPtr device = nullptr;
Microsoft::WRL::ComPtr endpoint_volume = nullptr;
std::shared_ptr raii_ptr(nullptr, [&](void*) {
endpoint_volume = nullptr;
device = nullptr;
enumerator = nullptr;
CoUninitialize();
});
HRESULT hr = CoCreateInstance(__uuidof(MMDeviceEnumerator), NULL, CLSCTX_ALL,
__uuidof(IMMDeviceEnumerator), (void**)enumerator.GetAddressOf());
if (hr != S_OK) {
std::cout << "CoCreateInstance error" << std::endl;
return;
}
hr = enumerator->GetDefaultAudioEndpoint(eRender, eConsole, device.GetAddressOf());
if (hr != S_OK) {
std::cout << "GetDefaultAudioEndpoint error" << std::endl;
return;
}
hr = device->Activate(__uuidof(IAudioEndpointVolume), CLSCTX_INPROC_SERVER,
NULL, (void**)endpoint_volume.GetAddressOf());
if (hr != S_OK) {
std::cout << "Activate error" << std::endl;
return;
}
endpoint_volume->SetMasterVolumeLevelScalar(volume, nullptr);
}
// 默认设备是不是静音
bool IsDefaultAudioDeviceMuted() {
CoInitializeEx(NULL, COINIT_MULTITHREADED);
Microsoft::WRL::ComPtr enumerator = nullptr;
Microsoft::WRL::ComPtr device = nullptr;
Microsoft::WRL::ComPtr endpoint_volume = nullptr;
std::shared_ptr raii_ptr(nullptr, [&](void*) {
endpoint_volume = nullptr;
device = nullptr;
enumerator = nullptr;
CoUninitialize();
});
HRESULT hr = CoCreateInstance(__uuidof(MMDeviceEnumerator), NULL, CLSCTX_ALL,
__uuidof(IMMDeviceEnumerator), (void**)enumerator.GetAddressOf());
if (hr != S_OK) {
std::cout << "CoCreateInstance error" << std::endl;
return false;
}
hr = enumerator->GetDefaultAudioEndpoint(eRender, eConsole, device.GetAddressOf());
if (hr != S_OK) {
std::cout << "GetDefaultAudioEndpoint error" << std::endl;
return false;
}
hr = device->Activate(__uuidof(IAudioEndpointVolume), CLSCTX_INPROC_SERVER,
NULL, (void**)endpoint_volume.GetAddressOf());
if (hr != S_OK) {
std::cout << "Activate error" << std::endl;
return false;
}
BOOL mute = false;
endpoint_volume->GetMute(&mute);
return mute;
}
// 设置默认设备是否为静音
void SetDefaultAudioDeviceMuted(bool mute) {
BOOL bmute = mute;
CoInitializeEx(NULL, COINIT_MULTITHREADED);
Microsoft::WRL::ComPtr enumerator = nullptr;
Microsoft::WRL::ComPtr device = nullptr;
Microsoft::WRL::ComPtr endpoint_volume = nullptr;
std::shared_ptr raii_ptr(nullptr, [&](void*) {
endpoint_volume = nullptr;
device = nullptr;
enumerator = nullptr;
CoUninitialize();
});
HRESULT hr = CoCreateInstance(__uuidof(MMDeviceEnumerator), NULL, CLSCTX_ALL,
__uuidof(IMMDeviceEnumerator), (void**)enumerator.GetAddressOf());
if (hr != S_OK) {
std::cout << "CoCreateInstance error" << std::endl;
return;
}
hr = enumerator->GetDefaultAudioEndpoint(eRender, eConsole, device.GetAddressOf());
if (hr != S_OK) {
std::cout << "GetDefaultAudioEndpoint error" << std::endl;
return;
}
hr = device->Activate(__uuidof(IAudioEndpointVolume), CLSCTX_INPROC_SERVER,
NULL, (void**)endpoint_volume.GetAddressOf());
if (hr != S_OK) {
std::cout << "Activate error" << std::endl;
return;
}
endpoint_volume->SetMute(bmute, nullptr);
}
二、获取扬声器的名字和id
void RequireAudioInfo() {
Microsoft::WRL::ComPtr device_enumerator = nullptr;
Microsoft::WRL::ComPtr device = nullptr;
Microsoft::WRL::ComPtr collection = nullptr;
LPWSTR current_device_id = NULL;
HRESULT hr = CoInitializeEx(NULL, COINIT_MULTITHREADED);
std::shared_ptr raii_ptr(nullptr, [&](void*) {
collection = nullptr;
device = nullptr;
device_enumerator = nullptr;
CoUninitialize();
});
hr = CoCreateInstance(__uuidof(MMDeviceEnumerator), NULL, CLSCTX_ALL,
__uuidof(IMMDeviceEnumerator), (void**)device_enumerator.GetAddressOf());
hr = device_enumerator->GetDefaultAudioEndpoint(eRender, eConsole, device.GetAddressOf());
hr = device_enumerator->EnumAudioEndpoints(eRender, DEVICE_STATE_ACTIVE, collection.GetAddressOf());
UINT count;
hr = collection->GetCount(&count);
device->GetId(¤t_device_id);
std::wcout << "current_device_id:" << current_device_id << std::endl;
CoTaskMemFree(current_device_id);
// 打印音频设备个数
std::cout << "count:" << count << std::endl;
for (int i = 0; i < count; ++i) {
IMMDevice* pEndpointDevice = NULL;
IDeviceTopology* deviceTopology = NULL;
IConnector* connector = NULL;
LPWSTR device_name = NULL;
LPWSTR device_id = NULL;
hr = collection->Item(i, &pEndpointDevice);
hr = pEndpointDevice->Activate(__uuidof(IDeviceTopology), CLSCTX_INPROC_SERVER, NULL,
(LPVOID*)&deviceTopology);
hr = deviceTopology->GetConnector(0, &connector);
hr = connector->GetConnectorIdConnectedTo(&device_name);
std::wcout << "device name:" << device_name << std::endl;
hr = pEndpointDevice->GetId(&device_id);
std::wcout << "device id:" << device_id << std::endl;
CoTaskMemFree(device_name);
CoTaskMemFree(device_id);
}
}
版权声明:本文内容由网络用户投稿,版权归原作者所有,本站不拥有其著作权,亦不承担相应法律责任。如果您发现本站中有涉嫌抄袭或描述失实的内容,请联系我们jiasou666@gmail.com 处理,核实后本网站将在24小时内删除侵权内容。
暂时没有评论,来抢沙发吧~